mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2025-01-01 02:36:12 -05:00
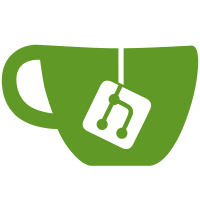
* Introduce _LAST_MODIFIED custom data entry that stores the last modified datetime of the database's custom data entries * Merge custom data from source database to target * Modify tests to be aware of _LAST_MODIFIED entry
181 lines
3.8 KiB
C++
181 lines
3.8 KiB
C++
/*
|
|
* Copyright (C) 2018 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include "CustomData.h"
|
|
#include "Clock.h"
|
|
|
|
#include "core/Global.h"
|
|
|
|
const QString CustomData::LastModified = "_LAST_MODIFIED";
|
|
|
|
CustomData::CustomData(QObject* parent)
|
|
: QObject(parent)
|
|
{
|
|
}
|
|
|
|
QList<QString> CustomData::keys() const
|
|
{
|
|
return m_data.keys();
|
|
}
|
|
|
|
bool CustomData::hasKey(const QString& key) const
|
|
{
|
|
return m_data.contains(key);
|
|
}
|
|
|
|
QString CustomData::value(const QString& key) const
|
|
{
|
|
return m_data.value(key);
|
|
}
|
|
|
|
bool CustomData::contains(const QString& key) const
|
|
{
|
|
return m_data.contains(key);
|
|
}
|
|
|
|
bool CustomData::containsValue(const QString& value) const
|
|
{
|
|
return asConst(m_data).values().contains(value);
|
|
}
|
|
|
|
void CustomData::set(const QString& key, const QString& value)
|
|
{
|
|
bool addAttribute = !m_data.contains(key);
|
|
bool changeValue = !addAttribute && (m_data.value(key) != value);
|
|
|
|
if (addAttribute) {
|
|
emit aboutToBeAdded(key);
|
|
}
|
|
|
|
if (addAttribute || changeValue) {
|
|
m_data.insert(key, value);
|
|
updateLastModified();
|
|
emit customDataModified();
|
|
}
|
|
|
|
if (addAttribute) {
|
|
emit added(key);
|
|
}
|
|
}
|
|
|
|
void CustomData::remove(const QString& key)
|
|
{
|
|
emit aboutToBeRemoved(key);
|
|
|
|
m_data.remove(key);
|
|
|
|
updateLastModified();
|
|
emit removed(key);
|
|
emit customDataModified();
|
|
}
|
|
|
|
void CustomData::rename(const QString& oldKey, const QString& newKey)
|
|
{
|
|
const bool containsOldKey = m_data.contains(oldKey);
|
|
const bool containsNewKey = m_data.contains(newKey);
|
|
Q_ASSERT(containsOldKey && !containsNewKey);
|
|
if (!containsOldKey || containsNewKey) {
|
|
return;
|
|
}
|
|
|
|
QString data = value(oldKey);
|
|
|
|
emit aboutToRename(oldKey, newKey);
|
|
|
|
m_data.remove(oldKey);
|
|
m_data.insert(newKey, data);
|
|
|
|
updateLastModified();
|
|
emit customDataModified();
|
|
emit renamed(oldKey, newKey);
|
|
}
|
|
|
|
void CustomData::copyDataFrom(const CustomData* other)
|
|
{
|
|
if (*this == *other) {
|
|
return;
|
|
}
|
|
|
|
emit aboutToBeReset();
|
|
|
|
m_data = other->m_data;
|
|
|
|
updateLastModified();
|
|
emit reset();
|
|
emit customDataModified();
|
|
}
|
|
|
|
QDateTime CustomData::getLastModified() const
|
|
{
|
|
if (m_data.contains(LastModified)) {
|
|
return Clock::parse(m_data.value(LastModified));
|
|
}
|
|
return {};
|
|
}
|
|
|
|
bool CustomData::operator==(const CustomData& other) const
|
|
{
|
|
return (m_data == other.m_data);
|
|
}
|
|
|
|
bool CustomData::operator!=(const CustomData& other) const
|
|
{
|
|
return (m_data != other.m_data);
|
|
}
|
|
|
|
void CustomData::clear()
|
|
{
|
|
emit aboutToBeReset();
|
|
|
|
m_data.clear();
|
|
|
|
emit reset();
|
|
emit customDataModified();
|
|
}
|
|
|
|
bool CustomData::isEmpty() const
|
|
{
|
|
return m_data.isEmpty();
|
|
}
|
|
|
|
int CustomData::size() const
|
|
{
|
|
return m_data.size();
|
|
}
|
|
|
|
int CustomData::dataSize() const
|
|
{
|
|
int size = 0;
|
|
|
|
QHashIterator<QString, QString> i(m_data);
|
|
while (i.hasNext()) {
|
|
i.next();
|
|
size += i.key().toUtf8().size() + i.value().toUtf8().size();
|
|
}
|
|
return size;
|
|
}
|
|
|
|
void CustomData::updateLastModified()
|
|
{
|
|
if (m_data.size() == 1 && m_data.contains(LastModified)) {
|
|
m_data.remove(LastModified);
|
|
return;
|
|
}
|
|
|
|
m_data.insert(LastModified, Clock::currentDateTimeUtc().toString());
|
|
}
|