mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2024-10-01 01:26:01 -04:00
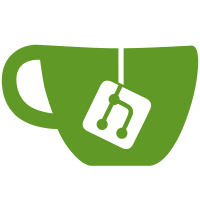
This has the advantage that they can be used without a running X server. Add methods to retrieve QPixmaps that are converted from the stored QImages and cached by QPixmapCache.
81 lines
2.9 KiB
C++
81 lines
2.9 KiB
C++
/*
|
|
* Copyright (C) 2010 Felix Geyer <debfx@fobos.de>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef KEEPASSX_KEEPASS2XMLWRITER_H
|
|
#define KEEPASSX_KEEPASS2XMLWRITER_H
|
|
|
|
#include <QtCore/QDateTime>
|
|
#include <QtCore/QXmlStreamWriter>
|
|
#include <QtGui/QColor>
|
|
#include <QtGui/QImage>
|
|
|
|
#include "core/Database.h"
|
|
#include "core/Entry.h"
|
|
#include "core/Group.h"
|
|
#include "core/TimeInfo.h"
|
|
#include "core/Uuid.h"
|
|
|
|
class Group;
|
|
class KeePass2RandomStream;
|
|
class Metadata;
|
|
|
|
class KeePass2XmlWriter
|
|
{
|
|
public:
|
|
KeePass2XmlWriter();
|
|
void writeDatabase(QIODevice* device, Database* db, KeePass2RandomStream* randomStream = 0);
|
|
void writeDatabase(const QString& filename, Database* db, KeePass2RandomStream* randomStream = 0);
|
|
bool error();
|
|
QString errorString();
|
|
|
|
private:
|
|
void writeMetadata();
|
|
void writeMemoryProtection();
|
|
void writeCustomIcons();
|
|
void writeIcon(const Uuid& uuid, const QImage& icon);
|
|
void writeCustomData();
|
|
void writeCustomDataItem(const QString& key, const QString& value);
|
|
void writeRoot();
|
|
void writeGroup(const Group* group);
|
|
void writeTimes(const TimeInfo& ti);
|
|
void writeDeletedObjects();
|
|
void writeDeletedObject(const DeletedObject& delObj);
|
|
void writeEntry(const Entry* entry);
|
|
void writeAutoType(const Entry* entry);
|
|
void writeAutoTypeAssoc(const AutoTypeAssociation& assoc);
|
|
void writeEntryHistory(const Entry* entry);
|
|
|
|
void writeString(const QString& qualifiedName, const QString& string);
|
|
void writeNumber(const QString& qualifiedName, int number);
|
|
void writeBool(const QString& qualifiedName, bool b);
|
|
void writeDateTime(const QString& qualifiedName, const QDateTime& dateTime);
|
|
void writeUuid(const QString& qualifiedName, const Uuid& uuid);
|
|
void writeUuid(const QString& qualifiedName, const Group* group);
|
|
void writeUuid(const QString& qualifiedName, const Entry* entry);
|
|
void writeBinary(const QString& qualifiedName, const QByteArray& ba);
|
|
void writeColor(const QString& qualifiedName, const QColor& color);
|
|
void writeTriState(const QString& qualifiedName, Group::TriState triState);
|
|
QString colorPartToString(int value);
|
|
|
|
QXmlStreamWriter m_xml;
|
|
Database* m_db;
|
|
Metadata* m_meta;
|
|
KeePass2RandomStream* m_randomStream;
|
|
};
|
|
|
|
#endif // KEEPASSX_KEEPASS2XMLWRITER_H
|