mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2025-07-16 03:29:29 -04:00
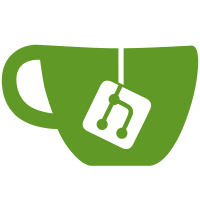
* SymmetricCipher: Fix Botan 3 build Botan commit 819cf8fe6278a19b8266f449228f02fc28a4f784 changed Botan::Cipher_Dir to be a scoped enumeration, so the users must be adapted. This change causes no issues with Botan 2 because normal enumeration values can also be referred to the same way scoped enumeration values are accessed. * Auto detect Botan3 * AsyncTask: Do not use `std::result_of` `std::result_of` was deprecated in C++17 and then it was subsequently removed in C++20. One could use `std::invoke_result_t`, but let Qt figure out the return type instead. * Collapse Botan2 and Botan3 find package into one * Update COPYING --------- Co-authored-by: Jonathan White <support@dmapps.us>
80 lines
2.7 KiB
C++
80 lines
2.7 KiB
C++
/*
|
|
* Copyright (C) 2017 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef KEEPASSXC_ASYNCTASK_HPP
|
|
#define KEEPASSXC_ASYNCTASK_HPP
|
|
|
|
#include <QFutureWatcher>
|
|
#include <QtConcurrent>
|
|
|
|
/**
|
|
* Asynchronously run computations outside the GUI thread.
|
|
*/
|
|
namespace AsyncTask
|
|
{
|
|
|
|
/**
|
|
* Wait for the given future without blocking the event loop.
|
|
*
|
|
* @param future future to wait for
|
|
* @return async task result
|
|
*/
|
|
template <typename T> T waitForFuture(QFuture<T> future)
|
|
{
|
|
QEventLoop loop;
|
|
QFutureWatcher<T> watcher;
|
|
QObject::connect(&watcher, SIGNAL(finished()), &loop, SLOT(quit()));
|
|
watcher.setFuture(future);
|
|
loop.exec();
|
|
return future.result();
|
|
}
|
|
|
|
/**
|
|
* Run a given task and wait for it to finish without blocking the event loop.
|
|
*
|
|
* @param task std::function object to run
|
|
* @return async task result
|
|
*/
|
|
template <typename FunctionObject> decltype(auto) runAndWaitForFuture(FunctionObject task)
|
|
{
|
|
return waitForFuture(QtConcurrent::run(task));
|
|
}
|
|
|
|
/**
|
|
* Run a given task then call the defined callback. Prevents event loop blocking and
|
|
* ensures the validity of the follow-on task through the context. If the context is
|
|
* deleted, the callback will not be processed preventing use after free errors.
|
|
*
|
|
* @param task std::function object to run
|
|
* @param context QObject responsible for calling this function
|
|
* @param callback std::function object to run after the task completes
|
|
*/
|
|
template <typename FunctionObject, typename FunctionObject2>
|
|
void runThenCallback(FunctionObject task, QObject* context, FunctionObject2 callback)
|
|
{
|
|
auto future = QtConcurrent::run(task);
|
|
auto watcher = new QFutureWatcher<decltype(future.result())>(context);
|
|
QObject::connect(watcher, &QFutureWatcherBase::finished, context, [=]() {
|
|
watcher->deleteLater();
|
|
callback(future.result());
|
|
});
|
|
watcher->setFuture(future);
|
|
}
|
|
|
|
}; // namespace AsyncTask
|
|
|
|
#endif // KEEPASSXC_ASYNCTASK_HPP
|