mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2025-07-12 01:29:38 -04:00
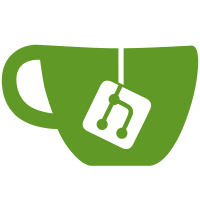
* Rename "Database Tags" to "Searches and Tags" * Separate searching for all entries and resetting the search * Support selecting multiple tags to search against * Fix using escaped quotes in search terms * Make tag searching more precise * Support `is:expired-#` to search for entries expiring within # days. Exclude recycled entries from expired search. * Don't list tags from entries that are recycled * Force hide tag auto-completion menu when tag editing widget is hidden. On rare occasions the focus out signal is not called when the tag view is hidden (entry edit is closed), this resolves that problem. * Remove spaces from before and after tags to prevent seemingly duplicate tags from being created. * Also fix some awkward signal/slot dances that were setup over time with the entry view and preview widget. Allow changing tags for multiple entries through context menu * Closes #8277 - show context menu with currently available tags in database and checks those that are set on one or more selected entries. When a tag is selected it is either set or unset on all entries depending on its checked state. * Add ability to save searches and recall them from the "Searches and Tags" view * Add ability to remove a tag from all entries from the "Searches and Tags" view * Cleanup tag handling and widgets
513 lines
11 KiB
C++
513 lines
11 KiB
C++
/*
|
|
* Copyright (C) 2010 Felix Geyer <debfx@fobos.de>
|
|
* Copyright (C) 2021 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include "Metadata.h"
|
|
|
|
#include "core/Clock.h"
|
|
#include "core/Entry.h"
|
|
#include "core/Group.h"
|
|
|
|
#include <QApplication>
|
|
#include <QCryptographicHash>
|
|
#include <QJsonDocument>
|
|
|
|
const int Metadata::DefaultHistoryMaxItems = 10;
|
|
const int Metadata::DefaultHistoryMaxSize = 6 * 1024 * 1024;
|
|
|
|
// Fallback icon for return by reference
|
|
static const Metadata::CustomIconData NULL_ICON{};
|
|
|
|
Metadata::Metadata(QObject* parent)
|
|
: ModifiableObject(parent)
|
|
, m_customData(new CustomData(this))
|
|
, m_updateDatetime(true)
|
|
{
|
|
init();
|
|
connect(m_customData, &CustomData::modified, this, &Metadata::modified);
|
|
}
|
|
|
|
void Metadata::init()
|
|
{
|
|
m_data.generator = QStringLiteral("KeePassXC");
|
|
m_data.maintenanceHistoryDays = 365;
|
|
m_data.masterKeyChangeRec = -1;
|
|
m_data.masterKeyChangeForce = -1;
|
|
m_data.historyMaxItems = DefaultHistoryMaxItems;
|
|
m_data.historyMaxSize = DefaultHistoryMaxSize;
|
|
m_data.recycleBinEnabled = true;
|
|
m_data.protectTitle = false;
|
|
m_data.protectUsername = false;
|
|
m_data.protectPassword = true;
|
|
m_data.protectUrl = false;
|
|
m_data.protectNotes = false;
|
|
|
|
QDateTime now = Clock::currentDateTimeUtc();
|
|
m_data.nameChanged = now;
|
|
m_data.descriptionChanged = now;
|
|
m_data.defaultUserNameChanged = now;
|
|
m_recycleBinChanged = now;
|
|
m_entryTemplatesGroupChanged = now;
|
|
m_masterKeyChanged = now;
|
|
m_settingsChanged = now;
|
|
}
|
|
|
|
void Metadata::clear()
|
|
{
|
|
init();
|
|
m_customIcons.clear();
|
|
m_customIconsOrder.clear();
|
|
m_customIconsHashes.clear();
|
|
m_customData->clear();
|
|
}
|
|
|
|
template <class P, class V> bool Metadata::set(P& property, const V& value)
|
|
{
|
|
if (property != value) {
|
|
property = value;
|
|
emitModified();
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
template <class P, class V> bool Metadata::set(P& property, const V& value, QDateTime& dateTime)
|
|
{
|
|
if (property != value) {
|
|
property = value;
|
|
if (m_updateDatetime) {
|
|
dateTime = Clock::currentDateTimeUtc();
|
|
}
|
|
emitModified();
|
|
return true;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
void Metadata::setUpdateDatetime(bool value)
|
|
{
|
|
m_updateDatetime = value;
|
|
}
|
|
|
|
void Metadata::copyAttributesFrom(const Metadata* other)
|
|
{
|
|
m_data = other->m_data;
|
|
}
|
|
|
|
QString Metadata::generator() const
|
|
{
|
|
return m_data.generator;
|
|
}
|
|
|
|
QString Metadata::name() const
|
|
{
|
|
return m_data.name;
|
|
}
|
|
|
|
QDateTime Metadata::nameChanged() const
|
|
{
|
|
return m_data.nameChanged;
|
|
}
|
|
|
|
QString Metadata::description() const
|
|
{
|
|
return m_data.description;
|
|
}
|
|
|
|
QDateTime Metadata::descriptionChanged() const
|
|
{
|
|
return m_data.descriptionChanged;
|
|
}
|
|
|
|
QString Metadata::defaultUserName() const
|
|
{
|
|
return m_data.defaultUserName;
|
|
}
|
|
|
|
QDateTime Metadata::defaultUserNameChanged() const
|
|
{
|
|
return m_data.defaultUserNameChanged;
|
|
}
|
|
|
|
int Metadata::maintenanceHistoryDays() const
|
|
{
|
|
return m_data.maintenanceHistoryDays;
|
|
}
|
|
|
|
QString Metadata::color() const
|
|
{
|
|
return m_data.color;
|
|
}
|
|
|
|
bool Metadata::protectTitle() const
|
|
{
|
|
return m_data.protectTitle;
|
|
}
|
|
|
|
bool Metadata::protectUsername() const
|
|
{
|
|
return m_data.protectUsername;
|
|
}
|
|
|
|
bool Metadata::protectPassword() const
|
|
{
|
|
return m_data.protectPassword;
|
|
}
|
|
|
|
bool Metadata::protectUrl() const
|
|
{
|
|
return m_data.protectUrl;
|
|
}
|
|
|
|
bool Metadata::protectNotes() const
|
|
{
|
|
return m_data.protectNotes;
|
|
}
|
|
|
|
const Metadata::CustomIconData& Metadata::customIcon(const QUuid& uuid) const
|
|
{
|
|
auto icon = m_customIcons.find(uuid);
|
|
Q_ASSERT(icon != m_customIcons.end());
|
|
if (icon == m_customIcons.end()) {
|
|
return NULL_ICON;
|
|
}
|
|
return icon.value();
|
|
}
|
|
|
|
bool Metadata::hasCustomIcon(const QUuid& uuid) const
|
|
{
|
|
return m_customIcons.contains(uuid);
|
|
}
|
|
|
|
QList<QUuid> Metadata::customIconsOrder() const
|
|
{
|
|
return m_customIconsOrder;
|
|
}
|
|
|
|
bool Metadata::recycleBinEnabled() const
|
|
{
|
|
return m_data.recycleBinEnabled;
|
|
}
|
|
|
|
Group* Metadata::recycleBin()
|
|
{
|
|
return m_recycleBin;
|
|
}
|
|
|
|
const Group* Metadata::recycleBin() const
|
|
{
|
|
return m_recycleBin;
|
|
}
|
|
|
|
QDateTime Metadata::recycleBinChanged() const
|
|
{
|
|
return m_recycleBinChanged;
|
|
}
|
|
|
|
const Group* Metadata::entryTemplatesGroup() const
|
|
{
|
|
return m_entryTemplatesGroup;
|
|
}
|
|
|
|
QDateTime Metadata::entryTemplatesGroupChanged() const
|
|
{
|
|
return m_entryTemplatesGroupChanged;
|
|
}
|
|
|
|
const Group* Metadata::lastSelectedGroup() const
|
|
{
|
|
return m_lastSelectedGroup;
|
|
}
|
|
|
|
const Group* Metadata::lastTopVisibleGroup() const
|
|
{
|
|
return m_lastTopVisibleGroup;
|
|
}
|
|
|
|
QDateTime Metadata::databaseKeyChanged() const
|
|
{
|
|
return m_masterKeyChanged;
|
|
}
|
|
|
|
int Metadata::databaseKeyChangeRec() const
|
|
{
|
|
return m_data.masterKeyChangeRec;
|
|
}
|
|
|
|
int Metadata::databaseKeyChangeForce() const
|
|
{
|
|
return m_data.masterKeyChangeForce;
|
|
}
|
|
|
|
int Metadata::historyMaxItems() const
|
|
{
|
|
return m_data.historyMaxItems;
|
|
}
|
|
|
|
int Metadata::historyMaxSize() const
|
|
{
|
|
return m_data.historyMaxSize;
|
|
}
|
|
|
|
CustomData* Metadata::customData()
|
|
{
|
|
return m_customData;
|
|
}
|
|
|
|
const CustomData* Metadata::customData() const
|
|
{
|
|
return m_customData;
|
|
}
|
|
|
|
void Metadata::setGenerator(const QString& value)
|
|
{
|
|
set(m_data.generator, value);
|
|
}
|
|
|
|
void Metadata::setName(const QString& value)
|
|
{
|
|
set(m_data.name, value, m_data.nameChanged);
|
|
}
|
|
|
|
void Metadata::setNameChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_data.nameChanged = value;
|
|
}
|
|
|
|
void Metadata::setDescription(const QString& value)
|
|
{
|
|
set(m_data.description, value, m_data.descriptionChanged);
|
|
}
|
|
|
|
void Metadata::setDescriptionChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_data.descriptionChanged = value;
|
|
}
|
|
|
|
void Metadata::setDefaultUserName(const QString& value)
|
|
{
|
|
set(m_data.defaultUserName, value, m_data.defaultUserNameChanged);
|
|
}
|
|
|
|
void Metadata::setDefaultUserNameChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_data.defaultUserNameChanged = value;
|
|
}
|
|
|
|
void Metadata::setMaintenanceHistoryDays(int value)
|
|
{
|
|
set(m_data.maintenanceHistoryDays, value);
|
|
}
|
|
|
|
void Metadata::setColor(const QString& value)
|
|
{
|
|
set(m_data.color, value);
|
|
}
|
|
|
|
void Metadata::setProtectTitle(bool value)
|
|
{
|
|
set(m_data.protectTitle, value);
|
|
}
|
|
|
|
void Metadata::setProtectUsername(bool value)
|
|
{
|
|
set(m_data.protectUsername, value);
|
|
}
|
|
|
|
void Metadata::setProtectPassword(bool value)
|
|
{
|
|
set(m_data.protectPassword, value);
|
|
}
|
|
|
|
void Metadata::setProtectUrl(bool value)
|
|
{
|
|
set(m_data.protectUrl, value);
|
|
}
|
|
|
|
void Metadata::setProtectNotes(bool value)
|
|
{
|
|
set(m_data.protectNotes, value);
|
|
}
|
|
|
|
void Metadata::addCustomIcon(const QUuid& uuid, const CustomIconData& iconData)
|
|
{
|
|
|
|
Q_ASSERT(!uuid.isNull());
|
|
Q_ASSERT(!m_customIcons.contains(uuid));
|
|
|
|
// remove all uuids to prevent duplicates in release mode
|
|
m_customIcons[uuid] = iconData;
|
|
m_customIconsOrder.removeAll(uuid);
|
|
m_customIconsOrder.append(uuid);
|
|
|
|
// Associate image hash to uuid
|
|
QByteArray hash = hashIcon(iconData.data);
|
|
m_customIconsHashes[hash] = uuid;
|
|
Q_ASSERT(m_customIcons.count() == m_customIconsOrder.count());
|
|
|
|
emitModified();
|
|
}
|
|
|
|
void Metadata::addCustomIcon(const QUuid& uuid,
|
|
const QByteArray& iconBytes,
|
|
const QString& name,
|
|
const QDateTime& lastModified)
|
|
{
|
|
addCustomIcon(uuid, {iconBytes, name, lastModified});
|
|
}
|
|
|
|
void Metadata::removeCustomIcon(const QUuid& uuid)
|
|
{
|
|
Q_ASSERT(!uuid.isNull());
|
|
Q_ASSERT(m_customIcons.contains(uuid));
|
|
|
|
// Remove hash record only if this is the same uuid
|
|
QByteArray hash = hashIcon(m_customIcons[uuid].data);
|
|
if (m_customIconsHashes.contains(hash) && m_customIconsHashes[hash] == uuid) {
|
|
m_customIconsHashes.remove(hash);
|
|
}
|
|
|
|
m_customIcons.remove(uuid);
|
|
m_customIconsOrder.removeAll(uuid);
|
|
Q_ASSERT(m_customIcons.count() == m_customIconsOrder.count());
|
|
dynamic_cast<Database*>(parent())->addDeletedObject(uuid);
|
|
emitModified();
|
|
}
|
|
|
|
QUuid Metadata::findCustomIcon(const QByteArray& candidate)
|
|
{
|
|
QByteArray hash = hashIcon(candidate);
|
|
return m_customIconsHashes.value(hash, QUuid());
|
|
}
|
|
|
|
void Metadata::copyCustomIcons(const QSet<QUuid>& iconList, const Metadata* otherMetadata)
|
|
{
|
|
for (const QUuid& uuid : iconList) {
|
|
Q_ASSERT(otherMetadata->hasCustomIcon(uuid));
|
|
|
|
if (!hasCustomIcon(uuid) && otherMetadata->hasCustomIcon(uuid)) {
|
|
addCustomIcon(uuid, otherMetadata->customIcon(uuid));
|
|
}
|
|
}
|
|
}
|
|
|
|
QByteArray Metadata::hashIcon(const QByteArray& iconData)
|
|
{
|
|
return QCryptographicHash::hash(iconData, QCryptographicHash::Md5);
|
|
}
|
|
|
|
void Metadata::setRecycleBinEnabled(bool value)
|
|
{
|
|
set(m_data.recycleBinEnabled, value);
|
|
}
|
|
|
|
void Metadata::setRecycleBin(Group* group)
|
|
{
|
|
set(m_recycleBin, group, m_recycleBinChanged);
|
|
}
|
|
|
|
void Metadata::setRecycleBinChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_recycleBinChanged = value;
|
|
}
|
|
|
|
void Metadata::setEntryTemplatesGroup(Group* group)
|
|
{
|
|
set(m_entryTemplatesGroup, group, m_entryTemplatesGroupChanged);
|
|
}
|
|
|
|
void Metadata::setEntryTemplatesGroupChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_entryTemplatesGroupChanged = value;
|
|
}
|
|
|
|
void Metadata::setLastSelectedGroup(Group* group)
|
|
{
|
|
set(m_lastSelectedGroup, group);
|
|
}
|
|
|
|
void Metadata::setLastTopVisibleGroup(Group* group)
|
|
{
|
|
set(m_lastTopVisibleGroup, group);
|
|
}
|
|
|
|
void Metadata::setDatabaseKeyChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_masterKeyChanged = value;
|
|
}
|
|
|
|
void Metadata::setMasterKeyChangeRec(int value)
|
|
{
|
|
set(m_data.masterKeyChangeRec, value);
|
|
}
|
|
|
|
void Metadata::setMasterKeyChangeForce(int value)
|
|
{
|
|
set(m_data.masterKeyChangeForce, value);
|
|
}
|
|
|
|
void Metadata::setHistoryMaxItems(int value)
|
|
{
|
|
set(m_data.historyMaxItems, value);
|
|
}
|
|
|
|
void Metadata::setHistoryMaxSize(int value)
|
|
{
|
|
set(m_data.historyMaxSize, value);
|
|
}
|
|
|
|
QDateTime Metadata::settingsChanged() const
|
|
{
|
|
return m_settingsChanged;
|
|
}
|
|
|
|
void Metadata::setSettingsChanged(const QDateTime& value)
|
|
{
|
|
Q_ASSERT(value.timeSpec() == Qt::UTC);
|
|
m_settingsChanged = value;
|
|
}
|
|
|
|
void Metadata::addSavedSearch(const QString& name, const QString& searchtext)
|
|
{
|
|
auto searches = savedSearches();
|
|
searches.insert(name, searchtext);
|
|
auto json = QJsonDocument::fromVariant(searches);
|
|
m_customData->set("KPXC_SavedSearch", json.toJson());
|
|
}
|
|
|
|
void Metadata::deleteSavedSearch(const QString& name)
|
|
{
|
|
auto searches = savedSearches();
|
|
searches.remove(name);
|
|
auto json = QJsonDocument::fromVariant(searches);
|
|
m_customData->set("KPXC_SavedSearch", json.toJson());
|
|
}
|
|
|
|
QVariantMap Metadata::savedSearches()
|
|
{
|
|
auto searches = m_customData->value("KPXC_SavedSearch");
|
|
auto json = QJsonDocument::fromJson(searches.toUtf8());
|
|
return json.toVariant().toMap();
|
|
}
|