mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2025-07-11 17:19:40 -04:00
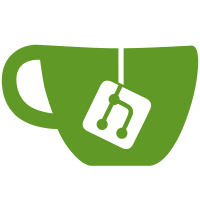
Externally opened attachments are now lifecycle-managed properly. The temporary files are created with stricter permissions and entirely random names (except for the file extension) to prevent meta data leakage. When the database is closed, the files are overwritten with random data and are also more reliably deleted than before. Changes to the temporary files are monitored and the user is asked if they want to save the changes back to the database (fixes #3130). KeePassXC does not keep a lock on any of the temporary files, resolving long-standing issues with applications such as Adobe Acrobat on Windows (fixes #5950, fixes #5839). Internally, attachments are copied less. The EntryAttachmentsWidget now only references EntryAttachments instead of owning a separate copy (which used to not be cleared properly under certain circumstances).
85 lines
2.4 KiB
C++
85 lines
2.4 KiB
C++
/*
|
|
* Copyright (C) 2017 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef KEEPASSX_DETAILSWIDGET_H
|
|
#define KEEPASSX_DETAILSWIDGET_H
|
|
|
|
#include "config-keepassx.h"
|
|
#include "gui/DatabaseWidget.h"
|
|
|
|
namespace Ui
|
|
{
|
|
class EntryPreviewWidget;
|
|
}
|
|
|
|
class QTextEdit;
|
|
|
|
class EntryPreviewWidget : public QWidget
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit EntryPreviewWidget(QWidget* parent = nullptr);
|
|
~EntryPreviewWidget() override;
|
|
|
|
public slots:
|
|
void setEntry(Entry* selectedEntry);
|
|
void setGroup(Group* selectedGroup);
|
|
void setDatabaseMode(DatabaseWidget::Mode mode);
|
|
void clear();
|
|
|
|
signals:
|
|
void errorOccurred(const QString& error);
|
|
void entryUrlActivated(Entry* entry);
|
|
|
|
private slots:
|
|
void updateEntryHeaderLine();
|
|
void updateEntryTotp();
|
|
void updateEntryGeneralTab();
|
|
void updateEntryAdvancedTab();
|
|
void updateEntryAutotypeTab();
|
|
void setPasswordVisible(bool state);
|
|
void setEntryNotesVisible(bool state);
|
|
void setGroupNotesVisible(bool state);
|
|
void setNotesVisible(QTextEdit* notesWidget, const QString& notes, bool state);
|
|
|
|
void updateGroupHeaderLine();
|
|
void updateGroupGeneralTab();
|
|
#if defined(WITH_XC_KEESHARE)
|
|
void updateGroupSharingTab();
|
|
#endif
|
|
|
|
void updateTotpLabel();
|
|
void updateTabIndexes();
|
|
void openEntryUrl();
|
|
|
|
private:
|
|
void removeTab(QTabWidget* tabWidget, QWidget* widget);
|
|
void setTabEnabled(QTabWidget* tabWidget, QWidget* widget, bool enabled);
|
|
|
|
static QString hierarchy(const Group* group, const QString& title);
|
|
|
|
const QScopedPointer<Ui::EntryPreviewWidget> m_ui;
|
|
bool m_locked;
|
|
QPointer<Entry> m_currentEntry;
|
|
QPointer<Group> m_currentGroup;
|
|
QTimer m_totpTimer;
|
|
quint8 m_selectedTabEntry;
|
|
quint8 m_selectedTabGroup;
|
|
};
|
|
|
|
#endif // KEEPASSX_DETAILSWIDGET_H
|