mirror of
https://github.com/keepassxreboot/keepassxc.git
synced 2025-03-12 17:16:43 -04:00
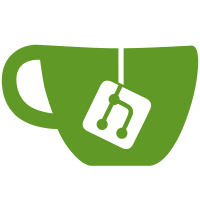
* Argon2 default parallelism settings were set to the number of threads on the computer. That is excessive on high cpu count computers.
70 lines
1.9 KiB
C++
70 lines
1.9 KiB
C++
/*
|
|
* Copyright (C) 2017 KeePassXC Team <team@keepassxc.org>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 or (at your option)
|
|
* version 3 of the License.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef KEEPASSX_ARGON2KDF_H
|
|
#define KEEPASSX_ARGON2KDF_H
|
|
|
|
#include "Kdf.h"
|
|
|
|
constexpr auto ARGON2_DEFAULT_VERSION = 0x13;
|
|
constexpr auto ARGON2_DEFAULT_ROUNDS = 10;
|
|
constexpr auto ARGON2_DEFAULT_MEMORY = 1 << 16;
|
|
constexpr auto ARGON2_DEFAULT_PARALLELISM = 2;
|
|
|
|
class Argon2Kdf : public Kdf
|
|
{
|
|
public:
|
|
enum class Type
|
|
{
|
|
Argon2d,
|
|
Argon2id
|
|
};
|
|
|
|
Argon2Kdf(Type type);
|
|
|
|
bool processParameters(const QVariantMap& p) override;
|
|
QVariantMap writeParameters() override;
|
|
bool transform(const QByteArray& raw, QByteArray& result) const override;
|
|
QSharedPointer<Kdf> clone() const override;
|
|
|
|
quint32 version() const;
|
|
bool setVersion(quint32 version);
|
|
Type type() const;
|
|
quint64 memory() const;
|
|
bool setMemory(quint64 kibibytes);
|
|
quint32 parallelism() const;
|
|
bool setParallelism(quint32 threads);
|
|
QString toString() const override;
|
|
|
|
int benchmark(int msec) const override;
|
|
|
|
static quint64 toMebibytes(quint64 kibibytes)
|
|
{
|
|
return kibibytes >> 10;
|
|
}
|
|
static quint64 toKibibytes(quint64 mebibits)
|
|
{
|
|
return mebibits << 10;
|
|
}
|
|
|
|
quint32 m_version;
|
|
quint64 m_memory;
|
|
quint32 m_parallelism;
|
|
};
|
|
|
|
#endif // KEEPASSX_ARGON2KDF_H
|