mirror of
https://github.com/GrapheneOS/hardened_malloc.git
synced 2024-06-29 08:02:11 +00:00
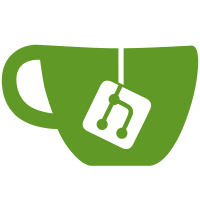
The stdint.h types don't cover 128-bit integers and the underscore makes them ill suited to usage in function suffixes. Instead, use the common naming style in the Linux kernel and elsewhere including the ChaCha8 implementation included here.
30 lines
619 B
C
30 lines
619 B
C
#ifndef UTIL_H
|
|
#define UTIL_H
|
|
|
|
#include <stdint.h>
|
|
#include <stdnoreturn.h>
|
|
|
|
#define likely(x) __builtin_expect(!!(x), 1)
|
|
#define unlikely(x) __builtin_expect(!!(x), 0)
|
|
|
|
#define COLD __attribute__((cold))
|
|
#define UNUSED __attribute__((unused))
|
|
#define EXPORT __attribute__((visibility("default")))
|
|
|
|
#define STRINGIFY(s) #s
|
|
#define ALIAS(f) __attribute__((alias(STRINGIFY(f))))
|
|
|
|
static inline int ffzl(long x) {
|
|
return __builtin_ffsl(~x);
|
|
}
|
|
|
|
COLD noreturn void fatal_error(const char *s);
|
|
|
|
typedef uint8_t u8;
|
|
typedef uint16_t u16;
|
|
typedef uint32_t u32;
|
|
typedef uint64_t u64;
|
|
typedef unsigned __int128 u128;
|
|
|
|
#endif
|