mirror of
https://github.com/benbusby/farside.git
synced 2025-03-21 14:26:42 -04:00
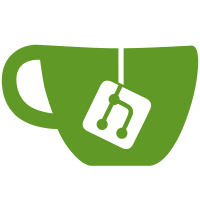
Introduces a new db key "<service>-previous" to track which instance was last selected for a particular service. This allows for filtering the list of available instances to exclude the instance that was last picked, to ensure a (slightly) more even distribution of traffic. There's still the possiblity of the following scenario, however: :service instances > 2 /:service request #1 -> instance #1 /:service request #2 -> instance #2 /:service request #3 -> instance #1 /:service request #4 -> instance #2 where there are many ignored instances for a particular service. One possible solution would be to implement the "<service>-previous" value to be a list, rather than a single value, and push to that list until only one element is left in the original "instance" array after filtering, and then delete the "<service>-previous" key.
70 lines
1.4 KiB
Elixir
70 lines
1.4 KiB
Elixir
defmodule FarsideTest do
|
|
@services_json Application.fetch_env!(:farside, :services_json)
|
|
|
|
use ExUnit.Case
|
|
use Plug.Test
|
|
|
|
alias Farside.Router
|
|
|
|
@opts Router.init([])
|
|
|
|
test "/" do
|
|
conn =
|
|
:get
|
|
|> conn("/", "")
|
|
|> Router.call(@opts)
|
|
|
|
assert conn.state == :sent
|
|
assert conn.status == 200
|
|
end
|
|
|
|
test "/ping" do
|
|
conn =
|
|
:get
|
|
|> conn("/ping", "")
|
|
|> Router.call(@opts)
|
|
|
|
assert conn.state == :sent
|
|
assert conn.status == 200
|
|
assert conn.resp_body == "PONG"
|
|
end
|
|
|
|
test "/:service" do
|
|
{:ok, file} = File.read(@services_json)
|
|
{:ok, service_list} = Poison.decode(file, as: [%{}])
|
|
|
|
service_names =
|
|
Enum.map(
|
|
service_list,
|
|
fn service -> service["type"] end
|
|
)
|
|
|
|
IO.puts("")
|
|
|
|
Enum.map(service_names, fn service_name ->
|
|
|
|
conn =
|
|
:get
|
|
|> conn("/#{service_name}", "")
|
|
|> Router.call(@opts)
|
|
|
|
first_redirect = elem(List.last(conn.resp_headers), 1)
|
|
IO.puts(" /#{service_name} (#1) -- #{first_redirect}")
|
|
assert conn.state == :set
|
|
assert conn.status == 302
|
|
|
|
|
|
conn =
|
|
:get
|
|
|> conn("/#{service_name}", "")
|
|
|> Router.call(@opts)
|
|
|
|
second_redirect = elem(List.last(conn.resp_headers), 1)
|
|
IO.puts(" /#{service_name} (#2) -- #{second_redirect}")
|
|
assert conn.state == :set
|
|
assert conn.status == 302
|
|
assert first_redirect != second_redirect
|
|
end)
|
|
end
|
|
end
|