mirror of
https://github.com/benbusby/farside.git
synced 2025-07-11 01:19:33 -04:00
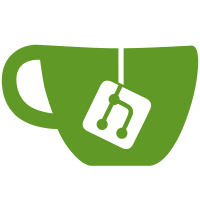
The project was rewritten from Elixir to Go, primarily because: - I don't write Elixir anymore and don't want to maintain a project in a language I no longer write - I already write Go for other projects, including my day job, so it's a safer bet for a project that I want to maintain long term - Go allows me to build portable executables that will make it easier for others to run farside on their own machines The Go version of Farsside also has a built in task to fetch the latest services{-full}.json file from the repo and ingest it, which makes running a farside server a lot simpler. It also automatically fetches the latest instance state from https://farside.link unless configured as a primary farside node, which will allow others to use farside without increasing traffic to all instances that are queried by farside (just to the farside node itself).
80 lines
1.7 KiB
Go
80 lines
1.7 KiB
Go
package server
|
|
|
|
import (
|
|
"io"
|
|
"log"
|
|
"net/http"
|
|
"net/http/httptest"
|
|
"net/url"
|
|
"os"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/benbusby/farside/db"
|
|
)
|
|
|
|
const breezewikiTestSite = "https://breezewikitest.com"
|
|
|
|
func TestMain(m *testing.M) {
|
|
err := db.InitializeDB()
|
|
if err != nil {
|
|
log.Fatalln("Failed to initialize database", err)
|
|
}
|
|
|
|
err = db.SetInstances("breezewiki", []string{breezewikiTestSite})
|
|
if err != nil {
|
|
log.Fatalln("Failed to set instances in db")
|
|
}
|
|
|
|
exitCode := m.Run()
|
|
|
|
_ = db.CloseDB()
|
|
os.Exit(exitCode)
|
|
}
|
|
|
|
func TestBaseRouting(t *testing.T) {
|
|
req := httptest.NewRequest(http.MethodGet, "/fandom.com", nil)
|
|
w := httptest.NewRecorder()
|
|
|
|
baseRouting(w, req)
|
|
|
|
res := w.Result()
|
|
defer res.Body.Close()
|
|
|
|
if res.StatusCode != http.StatusFound {
|
|
t.Fatalf("Incorrect resp code (%d) in base routing", res.StatusCode)
|
|
}
|
|
|
|
expectedHost, _ := url.Parse(breezewikiTestSite)
|
|
redirect, err := res.Location()
|
|
if err != nil {
|
|
t.Fatalf("Error retrieving direct from request: %v\n", err)
|
|
} else if redirect.Host != expectedHost.Host {
|
|
t.Fatalf("Incorrect redirect site -- expected: %s, actual: %s\n",
|
|
expectedHost.Host,
|
|
redirect.Host)
|
|
}
|
|
}
|
|
|
|
func TestJSRouting(t *testing.T) {
|
|
req := httptest.NewRequest(http.MethodGet, "/_/fandom.com", nil)
|
|
w := httptest.NewRecorder()
|
|
|
|
jsRouting(w, req)
|
|
|
|
res := w.Result()
|
|
defer res.Body.Close()
|
|
|
|
if res.StatusCode != http.StatusOK {
|
|
t.Fatalf("Incorrect resp code (%d) in base routing", res.StatusCode)
|
|
}
|
|
|
|
data, err := io.ReadAll(res.Body)
|
|
if err != nil {
|
|
t.Fatalf("Error reading response body: %v", err)
|
|
}
|
|
|
|
if !strings.Contains(string(data), breezewikiTestSite) {
|
|
t.Fatalf("%s not found in response body (%s)", breezewikiTestSite, string(data))
|
|
}
|
|
}
|