mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
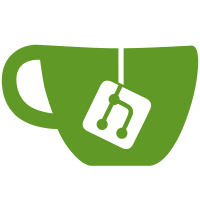
* build: correct toolchain order * build: gazelle-update-repos * build: use pregenerated proto for dependencies * update bazeldnf * deps: tpm simulator * Update Google trillian module * cli: add stamping as alternative build info source * bazel: add go_test wrappers, mark special tests and select testing deps * deps: add libvirt deps * deps: go-libvirt patches * deps: cloudflare circl patches * bazel: add go_test wrappers, mark special tests and select testing deps * bazel: keep gazelle overrides * bazel: cleanup bazelrc * bazel: switch CMakeLists.txt to use bazel * bazel: fix injection of version information via stamping * bazel: commit all build files * dev-docs: document bazel usage * deps: upgrade zig-cc for go 1.20 * bazel: update Perl for macOS arm64 & Linux arm64 support * bazel: use static perl toolchain for OpenSSL * bazel: use static protobuf (protoc) toolchain * deps: add git and go to nix deps Co-authored-by: Paul Meyer <49727155+katexochen@users.noreply.github.com>
54 lines
1.4 KiB
C++
54 lines
1.4 KiB
C++
#include <openssl/sha.h>
|
|
|
|
#include <cassert>
|
|
#include <iomanip>
|
|
#include <sstream>
|
|
#include <string>
|
|
|
|
// Use (void) to silent unused warnings.
|
|
#define assertm(exp, msg) assert(((void)msg, exp))
|
|
|
|
// From https://stackoverflow.com/a/2262447/7768383
|
|
bool simpleSHA256(const void* input, unsigned long length, unsigned char* md)
|
|
{
|
|
SHA256_CTX context;
|
|
if (!SHA256_Init(&context))
|
|
return false;
|
|
|
|
if (!SHA256_Update(&context, (unsigned char*)input, length))
|
|
return false;
|
|
|
|
if (!SHA256_Final(md, &context))
|
|
return false;
|
|
|
|
return true;
|
|
}
|
|
|
|
// Convert an byte array into a string
|
|
std::string fromByteArray(const unsigned char* data, unsigned long length)
|
|
{
|
|
std::stringstream shastr;
|
|
shastr << std::hex << std::setfill('0');
|
|
for (unsigned long i = 0; i < length; ++i)
|
|
{
|
|
shastr << std::setw(2) << static_cast<int>(data[i]);
|
|
}
|
|
|
|
return shastr.str();
|
|
}
|
|
|
|
std::string MESSAGE = "hello world";
|
|
std::string MESSAGE_HASH = "b94d27b9934d3e08a52e52d7da7dabfac484efe37a5380ee9088f7ace2efcde9";
|
|
|
|
int main(int argc, char* argv[])
|
|
{
|
|
unsigned char md[SHA256_DIGEST_LENGTH] = {};
|
|
|
|
assertm(simpleSHA256(static_cast<const void*>(MESSAGE.data()), MESSAGE.size(), md), "Failed to generate hash");
|
|
std::string hash = fromByteArray(md, SHA256_DIGEST_LENGTH);
|
|
|
|
assertm(hash == MESSAGE_HASH, "Unexpected message hash");
|
|
|
|
return 0;
|
|
}
|