mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
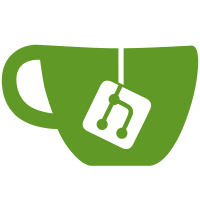
* terraform-provider: add usage example for Azure * terraform-provider: add usage example for AWS * terraform-provider: add usage example for GCP * terraform-provider: update usage example for Azure * terraform-provider: update generated documentation * docs: adjust creation on Azure and link to examples * terraform-provider: unify image in-/output (#2725) * terraform-provider: check for returned error when converting microservices * terraform-provider: use state values for outputs after creation * terraform-provider: ignore invalid upgrades (#2728) --------- Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> Co-authored-by: Thomas Tendyck <51411342+thomasten@users.noreply.github.com>
194 lines
6.6 KiB
Go
194 lines
6.6 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package provider
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"encoding/json"
|
|
"fmt"
|
|
"strconv"
|
|
|
|
"github.com/edgelesssys/constellation/v2/internal/api/attestationconfigapi"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/idkeydigest"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/measurements"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/variant"
|
|
"github.com/edgelesssys/constellation/v2/internal/config"
|
|
)
|
|
|
|
// naming schema:
|
|
// convertFromTf<type> : convert a terraform struct to a constellation struct
|
|
// convertToTf<type> : convert a constellation struct to a terraform struct
|
|
// terraform struct: used to parse the terraform state
|
|
// constellation struct: used to call the constellation API
|
|
|
|
// convertFromTfAttestationCfg converts the related terraform struct to a constellation attestation config.
|
|
func convertFromTfAttestationCfg(tfAttestation attestationAttribute, attestationVariant variant.Variant) (config.AttestationCfg, error) {
|
|
c11nMeasurements := make(measurements.M)
|
|
for strIdx, v := range tfAttestation.Measurements {
|
|
idx, err := strconv.ParseUint(strIdx, 10, 32)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
expectedBt, err := hex.DecodeString(v.Expected)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
var valOption measurements.MeasurementValidationOption
|
|
switch v.WarnOnly {
|
|
case true:
|
|
valOption = measurements.WarnOnly
|
|
case false:
|
|
valOption = measurements.Enforce
|
|
}
|
|
c11nMeasurements[uint32(idx)] = measurements.Measurement{
|
|
Expected: expectedBt,
|
|
ValidationOpt: valOption,
|
|
}
|
|
}
|
|
|
|
var attestationConfig config.AttestationCfg
|
|
switch attestationVariant {
|
|
case variant.AzureSEVSNP{}:
|
|
firmwareCfg, err := convertFromTfFirmwareCfg(tfAttestation.AzureSNPFirmwareSignerConfig)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("converting firmware signer config: %w", err)
|
|
}
|
|
|
|
var rootKey config.Certificate
|
|
if err := json.Unmarshal([]byte(tfAttestation.AMDRootKey), &rootKey); err != nil {
|
|
return nil, fmt.Errorf("unmarshalling root key: %w", err)
|
|
}
|
|
|
|
attestationConfig = &config.AzureSEVSNP{
|
|
Measurements: c11nMeasurements,
|
|
BootloaderVersion: newVersion(tfAttestation.BootloaderVersion),
|
|
TEEVersion: newVersion(tfAttestation.TEEVersion),
|
|
SNPVersion: newVersion(tfAttestation.SNPVersion),
|
|
MicrocodeVersion: newVersion(tfAttestation.MicrocodeVersion),
|
|
FirmwareSignerConfig: firmwareCfg,
|
|
AMDRootKey: rootKey,
|
|
}
|
|
case variant.AWSSEVSNP{}:
|
|
var rootKey config.Certificate
|
|
if err := json.Unmarshal([]byte(tfAttestation.AMDRootKey), &rootKey); err != nil {
|
|
return nil, fmt.Errorf("unmarshalling root key: %w", err)
|
|
}
|
|
|
|
attestationConfig = &config.AWSSEVSNP{
|
|
Measurements: c11nMeasurements,
|
|
BootloaderVersion: newVersion(tfAttestation.BootloaderVersion),
|
|
TEEVersion: newVersion(tfAttestation.TEEVersion),
|
|
SNPVersion: newVersion(tfAttestation.SNPVersion),
|
|
MicrocodeVersion: newVersion(tfAttestation.MicrocodeVersion),
|
|
AMDRootKey: rootKey,
|
|
}
|
|
case variant.GCPSEVES{}:
|
|
attestationConfig = &config.GCPSEVES{
|
|
Measurements: c11nMeasurements,
|
|
}
|
|
default:
|
|
return nil, fmt.Errorf("unknown attestation variant: %s", attestationVariant)
|
|
}
|
|
return attestationConfig, nil
|
|
}
|
|
|
|
// convertToTfAttestationCfg converts the constellation attestation config to the related terraform structs.
|
|
func convertToTfAttestation(attVar variant.Variant, snpVersions attestationconfigapi.SEVSNPVersionAPI) (tfAttestation attestationAttribute, err error) {
|
|
tfAttestation = attestationAttribute{
|
|
Variant: attVar.String(),
|
|
BootloaderVersion: snpVersions.Bootloader,
|
|
TEEVersion: snpVersions.TEE,
|
|
SNPVersion: snpVersions.SNP,
|
|
MicrocodeVersion: snpVersions.Microcode,
|
|
}
|
|
|
|
switch attVar {
|
|
case variant.AWSSEVSNP{}:
|
|
certStr, err := certAsString(config.DefaultForAWSSEVSNP().AMDRootKey)
|
|
if err != nil {
|
|
return tfAttestation, err
|
|
}
|
|
tfAttestation.AMDRootKey = certStr
|
|
|
|
case variant.AzureSEVSNP{}:
|
|
certStr, err := certAsString(config.DefaultForAzureSEVSNP().AMDRootKey)
|
|
if err != nil {
|
|
return tfAttestation, err
|
|
}
|
|
tfAttestation.AMDRootKey = certStr
|
|
|
|
firmwareCfg := config.DefaultForAzureSEVSNP().FirmwareSignerConfig
|
|
tfFirmwareCfg, err := convertToTfFirmwareCfg(firmwareCfg)
|
|
if err != nil {
|
|
return tfAttestation, err
|
|
}
|
|
tfAttestation.AzureSNPFirmwareSignerConfig = tfFirmwareCfg
|
|
case variant.GCPSEVES{}:
|
|
// no additional fields
|
|
default:
|
|
return tfAttestation, fmt.Errorf("unknown attestation variant: %s", attVar)
|
|
}
|
|
return tfAttestation, nil
|
|
}
|
|
|
|
func certAsString(cert config.Certificate) (string, error) {
|
|
certBytes, err := cert.MarshalJSON()
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
return string(certBytes), nil
|
|
}
|
|
|
|
// convertToTfFirmwareCfg converts the constellation firmware config to the terraform struct.
|
|
func convertToTfFirmwareCfg(firmwareCfg config.SNPFirmwareSignerConfig) (azureSnpFirmwareSignerConfigAttribute, error) {
|
|
keyDigestAny, err := firmwareCfg.AcceptedKeyDigests.MarshalYAML()
|
|
if err != nil {
|
|
return azureSnpFirmwareSignerConfigAttribute{}, err
|
|
}
|
|
keyDigest, ok := keyDigestAny.([]string)
|
|
if !ok {
|
|
return azureSnpFirmwareSignerConfigAttribute{}, fmt.Errorf("reading Accepted Key Digests: could not convert %T to []string", keyDigestAny)
|
|
}
|
|
return azureSnpFirmwareSignerConfigAttribute{
|
|
AcceptedKeyDigests: keyDigest,
|
|
EnforcementPolicy: firmwareCfg.EnforcementPolicy.String(),
|
|
MAAURL: firmwareCfg.MAAURL,
|
|
}, nil
|
|
}
|
|
|
|
// convertFromTfFirmwareCfg converts the terraform struct to a constellation firmware config.
|
|
func convertFromTfFirmwareCfg(tfFirmwareCfg azureSnpFirmwareSignerConfigAttribute) (config.SNPFirmwareSignerConfig, error) {
|
|
keyDigests, err := idkeydigest.UnmarshalHexString(tfFirmwareCfg.AcceptedKeyDigests)
|
|
if err != nil {
|
|
return config.SNPFirmwareSignerConfig{}, err
|
|
}
|
|
return config.SNPFirmwareSignerConfig{
|
|
AcceptedKeyDigests: keyDigests,
|
|
EnforcementPolicy: idkeydigest.EnforcePolicyFromString(tfFirmwareCfg.EnforcementPolicy),
|
|
MAAURL: tfFirmwareCfg.MAAURL,
|
|
}, nil
|
|
}
|
|
|
|
// convertToTfMeasurements converts the constellation measurements to the terraform struct.
|
|
func convertToTfMeasurements(m measurements.M) map[string]measurementAttribute {
|
|
tfMeasurements := map[string]measurementAttribute{}
|
|
for key, value := range m {
|
|
keyStr := strconv.FormatUint(uint64(key), 10)
|
|
tfMeasurements[keyStr] = measurementAttribute{
|
|
Expected: hex.EncodeToString(value.Expected),
|
|
WarnOnly: bool(value.ValidationOpt),
|
|
}
|
|
}
|
|
return tfMeasurements
|
|
}
|
|
|
|
func newVersion(v uint8) config.AttestationVersion {
|
|
return config.AttestationVersion{
|
|
Value: v,
|
|
}
|
|
}
|