mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-20 00:06:21 +00:00
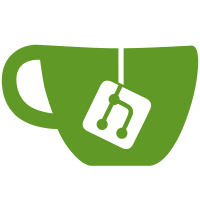
* Move `file`, `ssh` and `user` packages to internal * Rename `SSHKey` to `(ssh.)UserKey` * Rename KeyValue / Publickey to PublicKey * Rename SSH key file from "debugd" to "ssh-keys" * Add CreateSSHUsers function to Core * Call CreateSSHUsers users on first control-plane node, when defined in config Tests: * Make StubUserCreator add entries to /etc/passwd * Add NewLinuxUserManagerFake for unit tests * Add unit tests & adjust existing ones to changes
45 lines
894 B
Go
45 lines
894 B
Go
package ssh
|
|
|
|
import (
|
|
"github.com/edgelesssys/constellation/coordinator/pubapi/pubproto"
|
|
)
|
|
|
|
// FromProtoSlice converts a SSH UserKey definition from pubproto to the Go flavor.
|
|
func FromProtoSlice(input []*pubproto.SSHUserKey) []UserKey {
|
|
if input == nil {
|
|
return nil
|
|
}
|
|
|
|
output := make([]UserKey, 0)
|
|
|
|
for _, pair := range input {
|
|
singlePair := UserKey{
|
|
Username: pair.Username,
|
|
PublicKey: pair.PublicKey,
|
|
}
|
|
|
|
output = append(output, singlePair)
|
|
}
|
|
|
|
return output
|
|
}
|
|
|
|
// ToProtoSlice converts a SSH UserKey definition from Go to pubproto flavor.
|
|
func ToProtoSlice(input []*UserKey) []*pubproto.SSHUserKey {
|
|
if input == nil {
|
|
return nil
|
|
}
|
|
|
|
output := make([]*pubproto.SSHUserKey, 0)
|
|
for _, pair := range input {
|
|
singlePair := pubproto.SSHUserKey{
|
|
Username: pair.Username,
|
|
PublicKey: pair.PublicKey,
|
|
}
|
|
|
|
output = append(output, &singlePair)
|
|
}
|
|
|
|
return output
|
|
}
|