mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
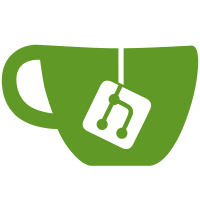
* terraform: enable creation of SEV-SNP VMs on GCP * variant: add SEV-SNP attestation variant * config: add SEV-SNP config options for GCP * measurements: add GCP SEV-SNP measurements * gcp: separate package for SEV-ES * attestation: add GCP SEV-SNP attestation logic * gcp: factor out common logic * choose: add GCP SEV-SNP * cli: add TF variable passthrough for GCP SEV-SNP variables * cli: support GCP SEV-SNP for `constellation verify` * Adjust usage of GCP SEV-SNP throughout codebase * ci: add GCP SEV-SNP * terraform-provider: support GCP SEV-SNP * docs: add GCP SEV-SNP reference * linter fixes * gcp: only run test with TPM simulator * gcp: remove nonsense test * Update cli/internal/cmd/verify.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update docs/docs/overview/clouds.md Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update terraform-provider-constellation/internal/provider/attestation_data_source_test.go Co-authored-by: Adrian Stobbe <stobbe.adrian@gmail.com> * linter fixes * terraform_provider: correctly pass down CC technology * config: mark attestationconfigapi as unimplemented * gcp: fix comments and typos * snp: use nonce and PK hash in SNP report * snp: ensure we never use ARK supplied by Issuer (#3025) * Make sure SNP ARK is always loaded from config, or fetched from AMD KDS * GCP: Set validator `reportData` correctly --------- Signed-off-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * attestationconfigapi: add GCP to uploading * snp: use correct cert Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * terraform-provider: enable fetching of attestation config values for GCP SEV-SNP * linter fixes --------- Signed-off-by: Daniel Weiße <dw@edgeless.systems> Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> Co-authored-by: Adrian Stobbe <stobbe.adrian@gmail.com>
82 lines
2.9 KiB
Go
82 lines
2.9 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package choose
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/edgelesssys/constellation/v2/internal/atls"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/aws/nitrotpm"
|
|
awssnp "github.com/edgelesssys/constellation/v2/internal/attestation/aws/snp"
|
|
azuresnp "github.com/edgelesssys/constellation/v2/internal/attestation/azure/snp"
|
|
azuretdx "github.com/edgelesssys/constellation/v2/internal/attestation/azure/tdx"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/azure/trustedlaunch"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/gcp/es"
|
|
gcpsnp "github.com/edgelesssys/constellation/v2/internal/attestation/gcp/snp"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/qemu"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/tdx"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/variant"
|
|
"github.com/edgelesssys/constellation/v2/internal/config"
|
|
)
|
|
|
|
// Issuer returns the issuer for the given variant.
|
|
func Issuer(attestationVariant variant.Variant, log attestation.Logger) (atls.Issuer, error) {
|
|
switch attestationVariant {
|
|
case variant.AWSSEVSNP{}:
|
|
return awssnp.NewIssuer(log), nil
|
|
case variant.AWSNitroTPM{}:
|
|
return nitrotpm.NewIssuer(log), nil
|
|
case variant.AzureTrustedLaunch{}:
|
|
return trustedlaunch.NewIssuer(log), nil
|
|
case variant.AzureSEVSNP{}:
|
|
return azuresnp.NewIssuer(log), nil
|
|
case variant.AzureTDX{}:
|
|
return azuretdx.NewIssuer(log), nil
|
|
case variant.GCPSEVES{}:
|
|
return es.NewIssuer(log), nil
|
|
case variant.GCPSEVSNP{}:
|
|
return gcpsnp.NewIssuer(log), nil
|
|
case variant.QEMUVTPM{}:
|
|
return qemu.NewIssuer(log), nil
|
|
case variant.QEMUTDX{}:
|
|
return tdx.NewIssuer(log), nil
|
|
case variant.Dummy{}:
|
|
return atls.NewFakeIssuer(variant.Dummy{}), nil
|
|
default:
|
|
return nil, fmt.Errorf("unknown attestation variant: %s", attestationVariant)
|
|
}
|
|
}
|
|
|
|
// Validator returns the validator for the given variant.
|
|
func Validator(cfg config.AttestationCfg, log attestation.Logger) (atls.Validator, error) {
|
|
switch cfg := cfg.(type) {
|
|
case *config.AWSSEVSNP:
|
|
return awssnp.NewValidator(cfg, log), nil
|
|
case *config.AWSNitroTPM:
|
|
return nitrotpm.NewValidator(cfg, log), nil
|
|
case *config.AzureTrustedLaunch:
|
|
return trustedlaunch.NewValidator(cfg, log), nil
|
|
case *config.AzureSEVSNP:
|
|
return azuresnp.NewValidator(cfg, log), nil
|
|
case *config.AzureTDX:
|
|
return azuretdx.NewValidator(cfg, log), nil
|
|
case *config.GCPSEVES:
|
|
return es.NewValidator(cfg, log)
|
|
case *config.GCPSEVSNP:
|
|
return gcpsnp.NewValidator(cfg, log)
|
|
case *config.QEMUVTPM:
|
|
return qemu.NewValidator(cfg, log), nil
|
|
case *config.QEMUTDX:
|
|
return tdx.NewValidator(cfg, log), nil
|
|
case *config.DummyCfg:
|
|
return atls.NewFakeValidator(variant.Dummy{}), nil
|
|
default:
|
|
return nil, fmt.Errorf("unknown attestation variant: %s", cfg.GetVariant())
|
|
}
|
|
}
|