mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
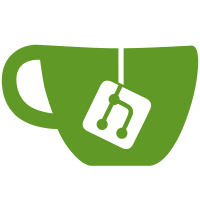
* There are now two attestation packages on azure. The issuer on the server side is created base on successfully querying the idkeydigest from the TPM. Fallback on err: Trusted Launch. * The bootstrapper's issuer choice is validated by the CLI's validator, which is created based on the local config. * Add "azureCVM" field to new "internal-config" cm. This field is populated by the bootstrapper. * Group attestation OIDs by CSP (#42) * Bootstrapper now uses IssuerWrapper type to pass the issuer (and some context info) to the initserver. * Introduce VMType package akin to cloudprovider. Used by IssuerWrapper. * Extend unittests. * Remove CSP specific attestation integration tests Co-authored-by: <dw@edgeless.systems> Signed-off-by: Otto Bittner <cobittner@posteo.net>
80 lines
2.0 KiB
Go
80 lines
2.0 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
|
|
Package oid defines OIDs for different CSPs. Currently this is used in attested TLS to distinguish the attestation documents.
|
|
OIDs beginning with 1.3.9900 are reserved and can be used without registration.
|
|
|
|
* The 1.3.9900.1 branch is reserved for placeholder values and testing.
|
|
|
|
* The 1.3.9900.2 branch is reserved for AWS.
|
|
|
|
* The 1.3.9900.3 branch is reserved for GCP.
|
|
|
|
* The 1.3.9900.4 branch is reserved for Azure.
|
|
|
|
* The 1.3.9900.5 branch is reserved for QEMU.
|
|
|
|
Deprecated OIDs should never be reused for different purposes.
|
|
Instead, new OIDs should be added in the appropriate branch at the next available index.
|
|
*/
|
|
package oid
|
|
|
|
import (
|
|
"encoding/asn1"
|
|
)
|
|
|
|
// Getter returns an ASN.1 Object Identifier.
|
|
type Getter interface {
|
|
OID() asn1.ObjectIdentifier
|
|
}
|
|
|
|
// Dummy OID for testing.
|
|
type Dummy struct{}
|
|
|
|
// OID returns the struct's object identifier.
|
|
func (Dummy) OID() asn1.ObjectIdentifier {
|
|
return asn1.ObjectIdentifier{1, 3, 9900, 1, 1}
|
|
}
|
|
|
|
// AWS holds the AWS OID.
|
|
type AWS struct{}
|
|
|
|
// OID returns the struct's object identifier.
|
|
func (AWS) OID() asn1.ObjectIdentifier {
|
|
return asn1.ObjectIdentifier{1, 3, 9900, 2, 1}
|
|
}
|
|
|
|
// GCP holds the GCP OID.
|
|
type GCP struct{}
|
|
|
|
// OID returns the struct's object identifier.
|
|
func (GCP) OID() asn1.ObjectIdentifier {
|
|
return asn1.ObjectIdentifier{1, 3, 9900, 3, 1}
|
|
}
|
|
|
|
// AzureSNP holds the OID for Azure SNP CVMs.
|
|
type AzureSNP struct{}
|
|
|
|
// OID returns the struct's object identifier.
|
|
func (AzureSNP) OID() asn1.ObjectIdentifier {
|
|
return asn1.ObjectIdentifier{1, 3, 9900, 4, 1}
|
|
}
|
|
|
|
// Azure holds the OID for Azure TrustedLaunch VMs.
|
|
type AzureTrustedLaunch struct{}
|
|
|
|
// OID returns the struct's object identifier.
|
|
func (AzureTrustedLaunch) OID() asn1.ObjectIdentifier {
|
|
return asn1.ObjectIdentifier{1, 3, 9900, 4, 2}
|
|
}
|
|
|
|
// QEMU holds the QEMU OID.
|
|
type QEMU struct{}
|
|
|
|
// OID returns the struct's object identifier.
|
|
func (QEMU) OID() asn1.ObjectIdentifier {
|
|
return asn1.ObjectIdentifier{1, 3, 9900, 5, 1}
|
|
}
|