mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-20 08:15:56 +00:00
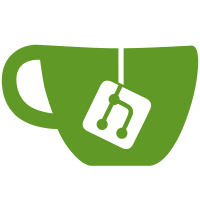
* Remove unused package * Add Go package docs to most packages Signed-off-by: Daniel Weiße <dw@edgeless.systems> Signed-off-by: Fabian Kammel <fk@edgeless.systems> Signed-off-by: Paul Meyer <49727155+katexochen@users.noreply.github.com> Co-authored-by: Paul Meyer <49727155+katexochen@users.noreply.github.com> Co-authored-by: Fabian Kammel <fk@edgeless.systems>
62 lines
1.7 KiB
Go
62 lines
1.7 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
// Package certificate provides functions to create a certificate request and matching private key.
|
|
package certificate
|
|
|
|
import (
|
|
"crypto/ecdsa"
|
|
"crypto/elliptic"
|
|
"crypto/rand"
|
|
"crypto/x509"
|
|
"crypto/x509/pkix"
|
|
"encoding/pem"
|
|
"net"
|
|
|
|
"k8s.io/kubernetes/cmd/kubeadm/app/constants"
|
|
)
|
|
|
|
const (
|
|
// CertificateFilename is the path to the kubelets certificate.
|
|
CertificateFilename = "/run/state/kubelet/pki/kubelet-client-crt.pem"
|
|
// KeyFilename is the path to the kubelets private key.
|
|
KeyFilename = "/run/state/kubelet/pki/kubelet-client-key.pem"
|
|
)
|
|
|
|
// GetKubeletCertificateRequest returns a certificate request and matching private key for the kubelet.
|
|
func GetKubeletCertificateRequest(nodeName string, ips []net.IP) (certificateRequest []byte, privateKey []byte, err error) {
|
|
csrTemplate := &x509.CertificateRequest{
|
|
Subject: pkix.Name{
|
|
Organization: []string{constants.NodesGroup},
|
|
CommonName: constants.NodesUserPrefix + nodeName,
|
|
},
|
|
IPAddresses: ips,
|
|
}
|
|
return GetCertificateRequest(csrTemplate)
|
|
}
|
|
|
|
// GetCertificateRequest returns a certificate request and matching private key.
|
|
func GetCertificateRequest(csrTemplate *x509.CertificateRequest) (certificateRequest []byte, privateKey []byte, err error) {
|
|
privK, err := ecdsa.GenerateKey(elliptic.P256(), rand.Reader)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
keyBytes, err := x509.MarshalECPrivateKey(privK)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
keyPem := pem.EncodeToMemory(&pem.Block{
|
|
Type: "EC PRIVATE KEY",
|
|
Bytes: keyBytes,
|
|
})
|
|
certificateRequest, err = x509.CreateCertificateRequest(rand.Reader, csrTemplate, privK)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
return certificateRequest, keyPem, nil
|
|
}
|