mirror of
https://github.com/edgelesssys/constellation.git
synced 2025-07-05 11:24:46 -04:00
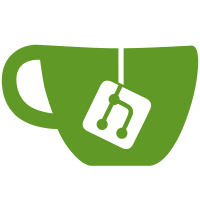
* rename to attestationconfigapi + put client and fetcher inside pkg * rename api/version to versionsapi and put fetcher + client inside pkg * rename AttestationConfigAPIFetcher to Fetcher
63 lines
1.5 KiB
Go
63 lines
1.5 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
package cmd
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/edgelesssys/constellation/v2/internal/api/attestationconfigapi"
|
|
"github.com/edgelesssys/constellation/v2/internal/staticupload"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
// newDeleteCmd creates the delete command.
|
|
func newDeleteCmd() *cobra.Command {
|
|
cmd := &cobra.Command{
|
|
Use: "delete",
|
|
Short: "delete a specific version from the config api",
|
|
RunE: runDelete,
|
|
}
|
|
cmd.Flags().StringP("version", "v", "", "Name of the version to delete (without .json suffix)")
|
|
must(enforceRequiredFlags(cmd, "version"))
|
|
return cmd
|
|
}
|
|
|
|
type deleteCmd struct {
|
|
attestationClient deleteClient
|
|
}
|
|
|
|
type deleteClient interface {
|
|
DeleteAzureSEVSNPVersion(ctx context.Context, versionStr string) error
|
|
}
|
|
|
|
func (d deleteCmd) delete(cmd *cobra.Command) error {
|
|
version, err := cmd.Flags().GetString("version")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return d.attestationClient.DeleteAzureSEVSNPVersion(cmd.Context(), version)
|
|
}
|
|
|
|
func runDelete(cmd *cobra.Command, _ []string) error {
|
|
cfg := staticupload.Config{
|
|
Bucket: awsBucket,
|
|
Region: awsRegion,
|
|
}
|
|
repo, closefn, err := attestationconfigapi.NewClient(cmd.Context(), cfg, []byte(cosignPwd), []byte(privateKey), false, log())
|
|
if err != nil {
|
|
return fmt.Errorf("create attestation client: %w", err)
|
|
}
|
|
defer func() {
|
|
if err := closefn(cmd.Context()); err != nil {
|
|
cmd.Printf("close client: %s\n", err.Error())
|
|
}
|
|
}()
|
|
deleteCmd := deleteCmd{
|
|
attestationClient: repo,
|
|
}
|
|
return deleteCmd.delete(cmd)
|
|
}
|