mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
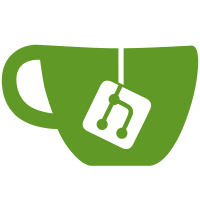
Co-authored-by: Malte Poll <mp@edgeless.systems> Co-authored-by: katexochen <katexochen@users.noreply.github.com> Co-authored-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Thomas Tendyck <tt@edgeless.systems> Co-authored-by: Benedict Schlueter <bs@edgeless.systems> Co-authored-by: leongross <leon.gross@rub.de> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com>
50 lines
1.5 KiB
Go
50 lines
1.5 KiB
Go
package config
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/edgelesssys/constellation/cli/file"
|
|
"github.com/edgelesssys/constellation/debugd/debugd/deploy"
|
|
"github.com/edgelesssys/constellation/debugd/ssh"
|
|
configc "github.com/edgelesssys/constellation/internal/config"
|
|
)
|
|
|
|
// CDBGConfig describes the constellation-cli config file and extends it with a new field "cdbg".
|
|
type CDBGConfig struct {
|
|
ConstellationDebugConfig ConstellationDebugdConfig `json:"cdbg"`
|
|
configc.Config ``
|
|
}
|
|
|
|
// ConstellationDebugdConfig is the cdbg specific configuration.
|
|
type ConstellationDebugdConfig struct {
|
|
AuthorizedKeys []ssh.SSHKey `json:"authorized_keys"`
|
|
CoordinatorPath string `json:"coordinator_path"`
|
|
SystemdUnits []deploy.SystemdUnit `json:"systemd_units,omitempty"`
|
|
}
|
|
|
|
// Default returns a struct with the default config.
|
|
func Default() *CDBGConfig {
|
|
return &CDBGConfig{
|
|
ConstellationDebugConfig: ConstellationDebugdConfig{
|
|
AuthorizedKeys: []ssh.SSHKey{},
|
|
CoordinatorPath: "coordinator",
|
|
SystemdUnits: []deploy.SystemdUnit{},
|
|
},
|
|
Config: *configc.Default(),
|
|
}
|
|
}
|
|
|
|
// FromFile returns a default config that has been merged with a config file.
|
|
// If name is empty, the defaults are returned.
|
|
func FromFile(fileHandler file.Handler, name string) (*CDBGConfig, error) {
|
|
conf := Default()
|
|
if name == "" {
|
|
return conf, nil
|
|
}
|
|
|
|
if err := fileHandler.ReadJSON(name, conf); err != nil {
|
|
return nil, fmt.Errorf("could not load config from file %s: %w", name, err)
|
|
}
|
|
return conf, nil
|
|
}
|