mirror of
https://github.com/edgelesssys/constellation.git
synced 2025-02-18 13:54:23 -05:00
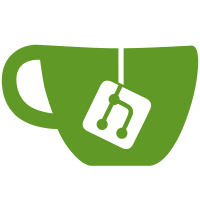
Co-authored-by: Malte Poll <mp@edgeless.systems> Co-authored-by: katexochen <katexochen@users.noreply.github.com> Co-authored-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Thomas Tendyck <tt@edgeless.systems> Co-authored-by: Benedict Schlueter <bs@edgeless.systems> Co-authored-by: leongross <leon.gross@rub.de> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com>
33 lines
884 B
Go
33 lines
884 B
Go
package gcp
|
|
|
|
import (
|
|
"context"
|
|
|
|
compute "cloud.google.com/go/compute/apiv1"
|
|
|
|
"github.com/googleapis/gax-go/v2"
|
|
computepb "google.golang.org/genproto/googleapis/cloud/compute/v1"
|
|
)
|
|
|
|
type instanceAPI interface {
|
|
Get(ctx context.Context, req *computepb.GetInstanceRequest, opts ...gax.CallOption) (*computepb.Instance, error)
|
|
List(ctx context.Context, req *computepb.ListInstancesRequest, opts ...gax.CallOption) InstanceIterator
|
|
SetMetadata(ctx context.Context, req *computepb.SetMetadataInstanceRequest, opts ...gax.CallOption) (*compute.Operation, error)
|
|
Close() error
|
|
}
|
|
|
|
type metadataAPI interface {
|
|
InstanceAttributeValue(attr string) (string, error)
|
|
ProjectID() (string, error)
|
|
Zone() (string, error)
|
|
InstanceName() (string, error)
|
|
}
|
|
|
|
type Operation interface {
|
|
Proto() *computepb.Operation
|
|
}
|
|
|
|
type InstanceIterator interface {
|
|
Next() (*computepb.Instance, error)
|
|
}
|