mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-20 16:26:19 +00:00
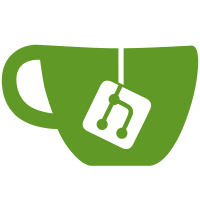
* Refactor disk-mapper recovery * Adapt constellation recover command to use new disk-mapper recovery API * Fix Cilium connectivity on rebooting nodes (#89) * Lower CoreDNS reschedule timeout to 10 seconds (#93) Signed-off-by: Daniel Weiße <dw@edgeless.systems>
51 lines
1.2 KiB
Go
51 lines
1.2 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package k8sapi
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/coreos/go-systemd/v22/dbus"
|
|
)
|
|
|
|
func startSystemdUnit(ctx context.Context, unit string) error {
|
|
conn, err := dbus.NewSystemdConnectionContext(ctx)
|
|
if err != nil {
|
|
return fmt.Errorf("establishing systemd connection: %w", err)
|
|
}
|
|
|
|
startChan := make(chan string)
|
|
if _, err := conn.StartUnitContext(ctx, unit, "replace", startChan); err != nil {
|
|
return fmt.Errorf("starting systemd unit %q: %w", unit, err)
|
|
}
|
|
|
|
// Wait for the enable to finish and actually check if it was
|
|
// successful or not.
|
|
result := <-startChan
|
|
|
|
switch result {
|
|
case "done":
|
|
return nil
|
|
|
|
default:
|
|
return fmt.Errorf("starting systemd unit %q failed: expected %v but received %v", unit, "done", result)
|
|
}
|
|
}
|
|
|
|
func enableSystemdUnit(ctx context.Context, unitPath string) error {
|
|
conn, err := dbus.NewSystemdConnectionContext(ctx)
|
|
if err != nil {
|
|
return fmt.Errorf("establishing systemd connection: %w", err)
|
|
}
|
|
|
|
if _, _, err := conn.EnableUnitFilesContext(ctx, []string{unitPath}, true, true); err != nil {
|
|
return fmt.Errorf("enabling systemd unit %q: %w", unitPath, err)
|
|
}
|
|
return nil
|
|
}
|