mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
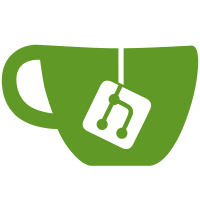
In the light of extending our eKMS support it will be helpful to have a tighter use of the word "KMS". KMS should refer to the actual component that manages keys. The keyservice, also called KMS in the constellation code, does not manage keys itself. It talks to a KMS backend, which in turn does the actual key management.
226 lines
7.3 KiB
Go
226 lines
7.3 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.28.1
|
|
// protoc v3.21.8
|
|
// source: keyservice.proto
|
|
|
|
package keyserviceproto
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type GetDataKeyRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
DataKeyId string `protobuf:"bytes,1,opt,name=data_key_id,json=dataKeyId,proto3" json:"data_key_id,omitempty"`
|
|
Length uint32 `protobuf:"varint,2,opt,name=length,proto3" json:"length,omitempty"`
|
|
}
|
|
|
|
func (x *GetDataKeyRequest) Reset() {
|
|
*x = GetDataKeyRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_keyservice_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *GetDataKeyRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetDataKeyRequest) ProtoMessage() {}
|
|
|
|
func (x *GetDataKeyRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_keyservice_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetDataKeyRequest.ProtoReflect.Descriptor instead.
|
|
func (*GetDataKeyRequest) Descriptor() ([]byte, []int) {
|
|
return file_keyservice_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *GetDataKeyRequest) GetDataKeyId() string {
|
|
if x != nil {
|
|
return x.DataKeyId
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GetDataKeyRequest) GetLength() uint32 {
|
|
if x != nil {
|
|
return x.Length
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type GetDataKeyResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
DataKey []byte `protobuf:"bytes,1,opt,name=data_key,json=dataKey,proto3" json:"data_key,omitempty"`
|
|
}
|
|
|
|
func (x *GetDataKeyResponse) Reset() {
|
|
*x = GetDataKeyResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_keyservice_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *GetDataKeyResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GetDataKeyResponse) ProtoMessage() {}
|
|
|
|
func (x *GetDataKeyResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_keyservice_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GetDataKeyResponse.ProtoReflect.Descriptor instead.
|
|
func (*GetDataKeyResponse) Descriptor() ([]byte, []int) {
|
|
return file_keyservice_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *GetDataKeyResponse) GetDataKey() []byte {
|
|
if x != nil {
|
|
return x.DataKey
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_keyservice_proto protoreflect.FileDescriptor
|
|
|
|
var file_keyservice_proto_rawDesc = []byte{
|
|
0x0a, 0x10, 0x6b, 0x65, 0x79, 0x73, 0x65, 0x72, 0x76, 0x69, 0x63, 0x65, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x12, 0x03, 0x6b, 0x6d, 0x73, 0x22, 0x4b, 0x0a, 0x11, 0x47, 0x65, 0x74, 0x44, 0x61,
|
|
0x74, 0x61, 0x4b, 0x65, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x1e, 0x0a, 0x0b,
|
|
0x64, 0x61, 0x74, 0x61, 0x5f, 0x6b, 0x65, 0x79, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x09, 0x64, 0x61, 0x74, 0x61, 0x4b, 0x65, 0x79, 0x49, 0x64, 0x12, 0x16, 0x0a, 0x06,
|
|
0x6c, 0x65, 0x6e, 0x67, 0x74, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x06, 0x6c, 0x65,
|
|
0x6e, 0x67, 0x74, 0x68, 0x22, 0x2f, 0x0a, 0x12, 0x47, 0x65, 0x74, 0x44, 0x61, 0x74, 0x61, 0x4b,
|
|
0x65, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x19, 0x0a, 0x08, 0x64, 0x61,
|
|
0x74, 0x61, 0x5f, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x07, 0x64, 0x61,
|
|
0x74, 0x61, 0x4b, 0x65, 0x79, 0x32, 0x44, 0x0a, 0x03, 0x41, 0x50, 0x49, 0x12, 0x3d, 0x0a, 0x0a,
|
|
0x47, 0x65, 0x74, 0x44, 0x61, 0x74, 0x61, 0x4b, 0x65, 0x79, 0x12, 0x16, 0x2e, 0x6b, 0x6d, 0x73,
|
|
0x2e, 0x47, 0x65, 0x74, 0x44, 0x61, 0x74, 0x61, 0x4b, 0x65, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x17, 0x2e, 0x6b, 0x6d, 0x73, 0x2e, 0x47, 0x65, 0x74, 0x44, 0x61, 0x74, 0x61,
|
|
0x4b, 0x65, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x42, 0x44, 0x5a, 0x42, 0x67,
|
|
0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x65, 0x64, 0x67, 0x65, 0x6c, 0x65,
|
|
0x73, 0x73, 0x73, 0x79, 0x73, 0x2f, 0x63, 0x6f, 0x6e, 0x73, 0x74, 0x65, 0x6c, 0x6c, 0x61, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x2f, 0x76, 0x32, 0x2f, 0x6b, 0x65, 0x79, 0x73, 0x65, 0x72, 0x76, 0x69, 0x63,
|
|
0x65, 0x2f, 0x6b, 0x65, 0x79, 0x73, 0x65, 0x72, 0x76, 0x69, 0x63, 0x65, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_keyservice_proto_rawDescOnce sync.Once
|
|
file_keyservice_proto_rawDescData = file_keyservice_proto_rawDesc
|
|
)
|
|
|
|
func file_keyservice_proto_rawDescGZIP() []byte {
|
|
file_keyservice_proto_rawDescOnce.Do(func() {
|
|
file_keyservice_proto_rawDescData = protoimpl.X.CompressGZIP(file_keyservice_proto_rawDescData)
|
|
})
|
|
return file_keyservice_proto_rawDescData
|
|
}
|
|
|
|
var file_keyservice_proto_msgTypes = make([]protoimpl.MessageInfo, 2)
|
|
var file_keyservice_proto_goTypes = []interface{}{
|
|
(*GetDataKeyRequest)(nil), // 0: kms.GetDataKeyRequest
|
|
(*GetDataKeyResponse)(nil), // 1: kms.GetDataKeyResponse
|
|
}
|
|
var file_keyservice_proto_depIdxs = []int32{
|
|
0, // 0: kms.API.GetDataKey:input_type -> kms.GetDataKeyRequest
|
|
1, // 1: kms.API.GetDataKey:output_type -> kms.GetDataKeyResponse
|
|
1, // [1:2] is the sub-list for method output_type
|
|
0, // [0:1] is the sub-list for method input_type
|
|
0, // [0:0] is the sub-list for extension type_name
|
|
0, // [0:0] is the sub-list for extension extendee
|
|
0, // [0:0] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_keyservice_proto_init() }
|
|
func file_keyservice_proto_init() {
|
|
if File_keyservice_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_keyservice_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*GetDataKeyRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_keyservice_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*GetDataKeyResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_keyservice_proto_rawDesc,
|
|
NumEnums: 0,
|
|
NumMessages: 2,
|
|
NumExtensions: 0,
|
|
NumServices: 1,
|
|
},
|
|
GoTypes: file_keyservice_proto_goTypes,
|
|
DependencyIndexes: file_keyservice_proto_depIdxs,
|
|
MessageInfos: file_keyservice_proto_msgTypes,
|
|
}.Build()
|
|
File_keyservice_proto = out.File
|
|
file_keyservice_proto_rawDesc = nil
|
|
file_keyservice_proto_goTypes = nil
|
|
file_keyservice_proto_depIdxs = nil
|
|
}
|