mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
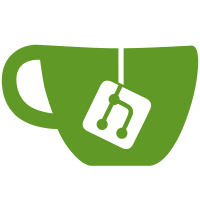
* terraform: add Azure marketplace variable Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * config: add Azure marketplace variable Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * cli: use Terraform variables from config Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * terraform: pass down marketplace variable * image: pad Azure images to 1GiB * terraform: add version attribute to marketplace image * semver: allow versions to be exported without prefix * cli: boolean var to use marketplace images * config: remove dive key * dev-docs: add instructions on how to use marketplace images * terraform: fix unit test * terraform: only fetch image for non-marketplace images * mpimage: refactor image selection Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [remove] increase minor version for image build Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * terraform: ignore changes to source_image_reference on upgrade * operator: add support for parsing Azure marketplace images Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * upgrade: fix imagefetcher call * docs: add info about azure marketplace * image: ensure more than 1GiB in size * image: test to pad to 2GiB * version: change back to v2.14.0-pre * image: GPT-conformant image size padding * [remove] increase version * mpimage: inline prefix func Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * ci: add marketplace image e2e test Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [remove] register workflow * ci: fix workflow name * ci: only allow azure test * cli: add marketplace image input to interface * cli: fix argument passing * version: roll back to v2.14.0 * ci: add force-flag support * Update docs/docs/overview/license.md * Update dev-docs/workflows/marketplace-images.md Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com> --------- Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com> Co-authored-by: Thomas Tendyck <51411342+thomasten@users.noreply.github.com>
81 lines
2.2 KiB
Go
81 lines
2.2 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package mpimage
|
|
|
|
import (
|
|
"fmt"
|
|
"net/url"
|
|
"strings"
|
|
|
|
"github.com/edgelesssys/constellation/v2/internal/cloud/cloudprovider"
|
|
"github.com/edgelesssys/constellation/v2/internal/constants"
|
|
"github.com/edgelesssys/constellation/v2/internal/semver"
|
|
)
|
|
|
|
// MarketplaceImage represents a CSP-agnostic marketplace image.
|
|
type MarketplaceImage interface {
|
|
URI() string
|
|
}
|
|
|
|
// NewFromURI returns a new MarketplaceImage for the given image URI.
|
|
func NewFromURI(uri string) (MarketplaceImage, error) {
|
|
u, err := url.Parse(uri)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if u.Scheme != constants.MarketplaceImageURIScheme {
|
|
return nil, fmt.Errorf("invalid scheme: %s", u.Scheme)
|
|
}
|
|
|
|
switch u.Host {
|
|
case cloudprovider.Azure.String():
|
|
ver, err := semver.New(u.Query().Get(constants.AzureMarketplaceImageVersionKey))
|
|
if err != nil {
|
|
return nil, fmt.Errorf("invalid image version: %w", err)
|
|
}
|
|
return NewAzureMarketplaceImage(ver), nil
|
|
default:
|
|
return nil, fmt.Errorf("invalid host: %s", u.Host)
|
|
}
|
|
}
|
|
|
|
// AzureMarketplaceImage represents an Azure marketplace image.
|
|
type AzureMarketplaceImage struct {
|
|
Publisher string
|
|
Offer string
|
|
SKU string
|
|
Version string
|
|
}
|
|
|
|
// NewAzureMarketplaceImage returns a new Constellation marketplace image for the given version.
|
|
func NewAzureMarketplaceImage(version semver.Semver) AzureMarketplaceImage {
|
|
return AzureMarketplaceImage{
|
|
Publisher: constants.AzureMarketplaceImagePublisher,
|
|
Offer: constants.AzureMarketplaceImageOffer,
|
|
SKU: constants.AzureMarketplaceImagePlan,
|
|
Version: strings.TrimPrefix(version.String(), "v"), // Azure requires X.Y.Z format
|
|
}
|
|
}
|
|
|
|
// URI returns the URI for the image.
|
|
func (i AzureMarketplaceImage) URI() string {
|
|
u := &url.URL{
|
|
Scheme: constants.MarketplaceImageURIScheme,
|
|
Host: cloudprovider.Azure.String(),
|
|
}
|
|
|
|
q := u.Query()
|
|
q.Set(constants.AzureMarketplaceImagePublisherKey, i.Publisher)
|
|
q.Set(constants.AzureMarketplaceImageOfferKey, i.Offer)
|
|
q.Set(constants.AzureMarketplaceImageSkuKey, i.SKU)
|
|
q.Set(constants.AzureMarketplaceImageVersionKey, i.Version)
|
|
u.RawQuery = q.Encode()
|
|
|
|
return u.String()
|
|
}
|