mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
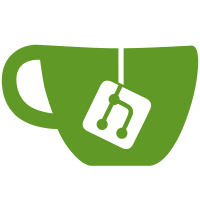
* re-use `ReadFromFile` in `CreateOrRead` Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [wip]: add constraints Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [wip] error formatting Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * wip Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * formatted error messages Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * state file validation Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * linter fixes Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * allow overriding the constraints Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * dont validate on read Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * add pre-create constraints Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [wip] Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * finish pre-init validation test Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * finish post-init validation Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * use state file validation in CLI Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * fix apply tests Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * Update internal/validation/errors.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * use transformator for tests * tidy * use empty check directly Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * Update cli/internal/state/state.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/state/state.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/state/state.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/state/state.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * conditional validation per CSP Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * tidy Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * fix rebase Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * add default case Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * validate state-file as last input Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> --------- Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com>
71 lines
1.8 KiB
Go
71 lines
1.8 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
/*
|
|
Package validation provides a unified document validation interface for use within the Constellation CLI.
|
|
|
|
It validates documents that specify a set of constraints on their content.
|
|
*/
|
|
package validation
|
|
|
|
import (
|
|
"errors"
|
|
)
|
|
|
|
// ErrStrategy is the strategy to use when encountering an error during validation.
|
|
type ErrStrategy int
|
|
|
|
const (
|
|
// EvaluateAll continues evaluating all constraints even if one is not satisfied.
|
|
EvaluateAll ErrStrategy = iota
|
|
// FailFast stops validation on the first error.
|
|
FailFast
|
|
)
|
|
|
|
// NewValidator creates a new Validator.
|
|
func NewValidator() *Validator {
|
|
return &Validator{}
|
|
}
|
|
|
|
// Validator validates documents.
|
|
type Validator struct{}
|
|
|
|
// Validatable is implemented by documents that can be validated.
|
|
// It returns a list of constraints that must be satisfied for the document to be valid.
|
|
type Validatable interface {
|
|
Constraints() []*Constraint
|
|
}
|
|
|
|
// ValidateOptions are the options to use when validating a document.
|
|
type ValidateOptions struct {
|
|
// ErrStrategy is the strategy to use when encountering an error during validation.
|
|
ErrStrategy ErrStrategy
|
|
// OverrideConstraints overrides the constraints to use for validation.
|
|
// If nil, the constraints returned by the document are used.
|
|
OverrideConstraints func() []*Constraint
|
|
}
|
|
|
|
// Validate validates a document using the given options.
|
|
func (v *Validator) Validate(doc Validatable, opts ValidateOptions) error {
|
|
var constraints func() []*Constraint
|
|
if opts.OverrideConstraints != nil {
|
|
constraints = opts.OverrideConstraints
|
|
} else {
|
|
constraints = doc.Constraints
|
|
}
|
|
|
|
var retErr error
|
|
for _, c := range constraints() {
|
|
if err := c.Satisfied(); err != nil {
|
|
if opts.ErrStrategy == FailFast {
|
|
return err
|
|
}
|
|
retErr = errors.Join(retErr, err)
|
|
}
|
|
}
|
|
return retErr
|
|
}
|