mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
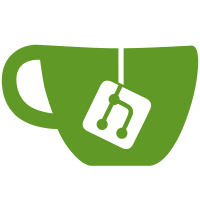
sed -i '1i/*\nCopyright (c) Edgeless Systems GmbH\n\nSPDX-License-Identifier: AGPL-3.0-only\n*/\n' `grep -rL --include='*.go' 'DO NOT EDIT'` gofumpt -w .
87 lines
2.0 KiB
Go
87 lines
2.0 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package gcp
|
|
|
|
import (
|
|
"context"
|
|
|
|
compute "cloud.google.com/go/compute/apiv1"
|
|
"cloud.google.com/go/compute/metadata"
|
|
"github.com/googleapis/gax-go/v2"
|
|
computepb "google.golang.org/genproto/googleapis/cloud/compute/v1"
|
|
)
|
|
|
|
type instanceClient struct {
|
|
*compute.InstancesClient
|
|
}
|
|
|
|
func (c *instanceClient) Close() error {
|
|
return c.InstancesClient.Close()
|
|
}
|
|
|
|
func (c *instanceClient) List(ctx context.Context, req *computepb.ListInstancesRequest,
|
|
opts ...gax.CallOption,
|
|
) InstanceIterator {
|
|
return c.InstancesClient.List(ctx, req)
|
|
}
|
|
|
|
type subnetworkClient struct {
|
|
*compute.SubnetworksClient
|
|
}
|
|
|
|
func (c *subnetworkClient) Close() error {
|
|
return c.SubnetworksClient.Close()
|
|
}
|
|
|
|
func (c *subnetworkClient) List(ctx context.Context, req *computepb.ListSubnetworksRequest,
|
|
opts ...gax.CallOption,
|
|
) SubnetworkIterator {
|
|
return c.SubnetworksClient.List(ctx, req)
|
|
}
|
|
|
|
func (c *subnetworkClient) Get(ctx context.Context, req *computepb.GetSubnetworkRequest,
|
|
opts ...gax.CallOption,
|
|
) (*computepb.Subnetwork, error) {
|
|
return c.SubnetworksClient.Get(ctx, req)
|
|
}
|
|
|
|
type forwardingRulesClient struct {
|
|
*compute.ForwardingRulesClient
|
|
}
|
|
|
|
func (c *forwardingRulesClient) Close() error {
|
|
return c.ForwardingRulesClient.Close()
|
|
}
|
|
|
|
func (c *forwardingRulesClient) List(ctx context.Context, req *computepb.ListForwardingRulesRequest,
|
|
opts ...gax.CallOption,
|
|
) ForwardingRuleIterator {
|
|
return c.ForwardingRulesClient.List(ctx, req)
|
|
}
|
|
|
|
type metadataClient struct{}
|
|
|
|
func (c *metadataClient) InstanceAttributeValue(attr string) (string, error) {
|
|
return metadata.InstanceAttributeValue(attr)
|
|
}
|
|
|
|
func (c *metadataClient) ProjectID() (string, error) {
|
|
return metadata.ProjectID()
|
|
}
|
|
|
|
func (c *metadataClient) Zone() (string, error) {
|
|
return metadata.Zone()
|
|
}
|
|
|
|
func (c *metadataClient) InstanceName() (string, error) {
|
|
return metadata.InstanceName()
|
|
}
|
|
|
|
func (c *metadataClient) ProjectAttributeValue(attr string) (string, error) {
|
|
return metadata.ProjectAttributeValue(attr)
|
|
}
|