mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-20 16:26:19 +00:00
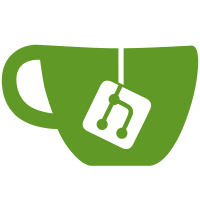
Co-authored-by: Malte Poll <mp@edgeless.systems> Co-authored-by: katexochen <katexochen@users.noreply.github.com> Co-authored-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Thomas Tendyck <tt@edgeless.systems> Co-authored-by: Benedict Schlueter <bs@edgeless.systems> Co-authored-by: leongross <leon.gross@rub.de> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com>
53 lines
1.4 KiB
Go
53 lines
1.4 KiB
Go
//go:build azure
|
|
// +build azure
|
|
|
|
package azure
|
|
|
|
import (
|
|
"encoding/json"
|
|
"testing"
|
|
|
|
"github.com/edgelesssys/constellation/coordinator/attestation/vtpm"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestAttestation(t *testing.T) {
|
|
assert := assert.New(t)
|
|
require := require.New(t)
|
|
|
|
issuer := NewIssuer()
|
|
validator := NewValidator(map[uint32][]byte{}) // TODO: check for list of expected Azure PCRs
|
|
|
|
nonce := []byte{2, 3, 4}
|
|
challenge := []byte("Constellation")
|
|
|
|
attDocRaw, err := issuer.Issue(challenge, nonce)
|
|
assert.NoError(err)
|
|
|
|
var attDoc vtpm.AttestationDocument
|
|
err = json.Unmarshal(attDocRaw, &attDoc)
|
|
require.NoError(err)
|
|
assert.Equal(challenge, attDoc.UserData)
|
|
originalPCR := attDoc.Attestation.Quotes[1].Pcrs.Pcrs[uint32(vtpm.PCRIndexOwnerID)]
|
|
|
|
out, err := validator.Validate(attDocRaw, nonce)
|
|
require.NoError(err)
|
|
assert.Equal(challenge, out)
|
|
|
|
// Mark node as intialized. We should still be abe to validate
|
|
assert.NoError(vtpm.MarkNodeAsInitialized(vtpm.OpenVTPM, []byte("Test"), []byte("Nonce")))
|
|
|
|
attDocRaw, err = issuer.Issue(challenge, nonce)
|
|
assert.NoError(err)
|
|
|
|
// Make sure the PCR changed
|
|
err = json.Unmarshal(attDocRaw, &attDoc)
|
|
require.NoError(err)
|
|
assert.NotEqual(originalPCR, attDoc.Attestation.Quotes[1].Pcrs.Pcrs[uint32(vtpm.PCRIndexOwnerID)])
|
|
|
|
out, err = validator.Validate(attDocRaw, nonce)
|
|
require.NoError(err)
|
|
assert.Equal(challenge, out)
|
|
}
|