mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
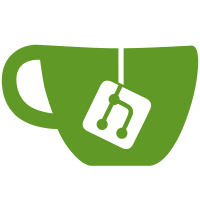
sed -i '1i/*\nCopyright (c) Edgeless Systems GmbH\n\nSPDX-License-Identifier: AGPL-3.0-only\n*/\n' `grep -rL --include='*.go' 'DO NOT EDIT'` gofumpt -w .
49 lines
1.0 KiB
Go
49 lines
1.0 KiB
Go
//go:build !disable_tpm_simulator
|
|
|
|
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package simulator
|
|
|
|
import (
|
|
"io"
|
|
|
|
"github.com/google/go-tpm-tools/simulator"
|
|
)
|
|
|
|
// OpenSimulatedTPM returns a simulated TPM device.
|
|
func OpenSimulatedTPM() (io.ReadWriteCloser, error) {
|
|
return simulator.Get()
|
|
}
|
|
|
|
// NewSimulatedTPMOpenFunc returns a TPMOpenFunc that opens a simulated TPM.
|
|
func NewSimulatedTPMOpenFunc() (func() (io.ReadWriteCloser, error), io.Closer) {
|
|
tpm, err := OpenSimulatedTPM()
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return func() (io.ReadWriteCloser, error) {
|
|
return &simulatedTPM{tpm}, nil
|
|
}, tpm
|
|
}
|
|
|
|
type simulatedTPM struct {
|
|
openSimulatedTPM io.ReadWriteCloser
|
|
}
|
|
|
|
func (t *simulatedTPM) Read(p []byte) (int, error) {
|
|
return t.openSimulatedTPM.Read(p)
|
|
}
|
|
|
|
func (t *simulatedTPM) Write(p []byte) (int, error) {
|
|
return t.openSimulatedTPM.Write(p)
|
|
}
|
|
|
|
func (t *simulatedTPM) Close() error {
|
|
// never close the underlying simulated TPM to allow calling the TPMOpenFunc again
|
|
return nil
|
|
}
|