mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
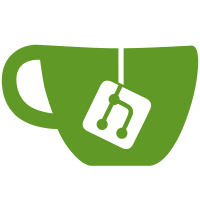
In the light of extending our eKMS support it will be helpful to have a tighter use of the word "KMS". KMS should refer to the actual component that manages keys. The keyservice, also called KMS in the constellation code, does not manage keys itself. It talks to a KMS backend, which in turn does the actual key management.
33 lines
1.1 KiB
Go
33 lines
1.1 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package kms
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
)
|
|
|
|
// CloudKMS enables using cloud base Key Management Services.
|
|
type CloudKMS interface {
|
|
// CreateKEK creates a new KEK with the given key material, if provided. If successful, the key can be referenced by keyID in the KMS in accordance to the policy.
|
|
CreateKEK(ctx context.Context, keyID string, kek []byte) error
|
|
// GetDEK returns the DEK for dekID and kekID from the KMS.
|
|
// If the DEK does not exist, a new one is created and saved to storage.
|
|
GetDEK(ctx context.Context, kekID string, dekID string, dekSize int) ([]byte, error)
|
|
}
|
|
|
|
// Storage provides an abstract interface for the storage backend used for DEKs.
|
|
type Storage interface {
|
|
// Get returns a DEK from the storage by key ID. If the DEK does not exist, returns storage.ErrDEKUnset.
|
|
Get(context.Context, string) ([]byte, error)
|
|
// Put saves a DEK to the storage by key ID.
|
|
Put(context.Context, string, []byte) error
|
|
}
|
|
|
|
// ErrKEKUnknown is an error raised by unknown KEK in the KMS.
|
|
var ErrKEKUnknown = errors.New("requested KEK not found")
|