mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
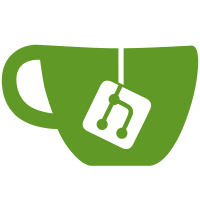
* terraform: enable creation of SEV-SNP VMs on GCP * variant: add SEV-SNP attestation variant * config: add SEV-SNP config options for GCP * measurements: add GCP SEV-SNP measurements * gcp: separate package for SEV-ES * attestation: add GCP SEV-SNP attestation logic * gcp: factor out common logic * choose: add GCP SEV-SNP * cli: add TF variable passthrough for GCP SEV-SNP variables * cli: support GCP SEV-SNP for `constellation verify` * Adjust usage of GCP SEV-SNP throughout codebase * ci: add GCP SEV-SNP * terraform-provider: support GCP SEV-SNP * docs: add GCP SEV-SNP reference * linter fixes * gcp: only run test with TPM simulator * gcp: remove nonsense test * Update cli/internal/cmd/verify.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update docs/docs/overview/clouds.md Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update terraform-provider-constellation/internal/provider/attestation_data_source_test.go Co-authored-by: Adrian Stobbe <stobbe.adrian@gmail.com> * linter fixes * terraform_provider: correctly pass down CC technology * config: mark attestationconfigapi as unimplemented * gcp: fix comments and typos * snp: use nonce and PK hash in SNP report * snp: ensure we never use ARK supplied by Issuer (#3025) * Make sure SNP ARK is always loaded from config, or fetched from AMD KDS * GCP: Set validator `reportData` correctly --------- Signed-off-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * attestationconfigapi: add GCP to uploading * snp: use correct cert Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * terraform-provider: enable fetching of attestation config values for GCP SEV-SNP * linter fixes --------- Signed-off-by: Daniel Weiße <dw@edgeless.systems> Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> Co-authored-by: Adrian Stobbe <stobbe.adrian@gmail.com>
70 lines
1.9 KiB
Go
70 lines
1.9 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package gcp
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
"errors"
|
|
"io"
|
|
|
|
"cloud.google.com/go/compute/metadata"
|
|
"github.com/google/go-tpm-tools/proto/attest"
|
|
)
|
|
|
|
// GCEInstanceInfo fetches VM metadata used for attestation from the GCE Metadata API.
|
|
func GCEInstanceInfo(client gcpMetadataClient) func(context.Context, io.ReadWriteCloser, []byte) ([]byte, error) {
|
|
// Ideally we would want to use the endorsement public key certificate
|
|
// However, this is not available on GCE instances
|
|
// Workaround: Provide ShieldedVM instance info
|
|
// The attestating party can request the VMs signing key using Google's API
|
|
return func(context.Context, io.ReadWriteCloser, []byte) ([]byte, error) {
|
|
projectID, err := client.ProjectID()
|
|
if err != nil {
|
|
return nil, errors.New("unable to fetch projectID")
|
|
}
|
|
zone, err := client.Zone()
|
|
if err != nil {
|
|
return nil, errors.New("unable to fetch zone")
|
|
}
|
|
instanceName, err := client.InstanceName()
|
|
if err != nil {
|
|
return nil, errors.New("unable to fetch instance name")
|
|
}
|
|
|
|
return json.Marshal(&attest.GCEInstanceInfo{
|
|
Zone: zone,
|
|
ProjectId: projectID,
|
|
InstanceName: instanceName,
|
|
})
|
|
}
|
|
}
|
|
|
|
type gcpMetadataClient interface {
|
|
ProjectID() (string, error)
|
|
InstanceName() (string, error)
|
|
Zone() (string, error)
|
|
}
|
|
|
|
// A MetadataClient fetches metadata from the GCE Metadata API.
|
|
type MetadataClient struct{}
|
|
|
|
// ProjectID returns the project ID of the GCE instance.
|
|
func (c MetadataClient) ProjectID() (string, error) {
|
|
return metadata.ProjectID()
|
|
}
|
|
|
|
// InstanceName returns the instance name of the GCE instance.
|
|
func (c MetadataClient) InstanceName() (string, error) {
|
|
return metadata.InstanceName()
|
|
}
|
|
|
|
// Zone returns the zone the GCE instance is located in.
|
|
func (c MetadataClient) Zone() (string, error) {
|
|
return metadata.Zone()
|
|
}
|