mirror of
https://github.com/edgelesssys/constellation.git
synced 2025-07-11 09:29:31 -04:00
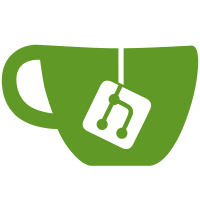
Run with: constellation upgrade execute --helm. This will only upgrade the helm charts. No config is needed. Upgrades are implemented via helm's upgrade action, i.e. they automatically roll back if something goes wrong. Releases could still be managed via helm, even after an upgrade with constellation has been done. Currently not user facing as CRD/CR backups are still in progress. These backups should be automatically created and saved to the user's disk as updates may delete CRs. This happens implicitly through CRD upgrades, which are part of microservice upgrades.
106 lines
3.3 KiB
Go
106 lines
3.3 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package cmd
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"time"
|
|
|
|
"github.com/edgelesssys/constellation/v2/cli/internal/cloudcmd"
|
|
"github.com/edgelesssys/constellation/v2/internal/attestation/measurements"
|
|
"github.com/edgelesssys/constellation/v2/internal/config"
|
|
"github.com/edgelesssys/constellation/v2/internal/file"
|
|
"github.com/edgelesssys/constellation/v2/internal/image"
|
|
"github.com/spf13/afero"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
func newUpgradeExecuteCmd() *cobra.Command {
|
|
cmd := &cobra.Command{
|
|
Use: "execute",
|
|
Short: "Execute an upgrade of a Constellation cluster",
|
|
Long: "Execute an upgrade of a Constellation cluster by applying the chosen configuration.",
|
|
Args: cobra.NoArgs,
|
|
RunE: runUpgradeExecute,
|
|
}
|
|
|
|
cmd.Flags().Bool("helm", false, "Execute helm upgrade. This feature is still in development an may change without anounncement. Upgrades all helm charts deployed during constellation-init.")
|
|
cmd.Flags().Duration("timeout", 3*time.Minute, "Change helm upgrade timeout. This feature is still in development an may change without anounncement. Might be useful for slow connections or big clusters.")
|
|
if err := cmd.Flags().MarkHidden("helm"); err != nil {
|
|
panic(err)
|
|
}
|
|
if err := cmd.Flags().MarkHidden("timeout"); err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
return cmd
|
|
}
|
|
|
|
func runUpgradeExecute(cmd *cobra.Command, args []string) error {
|
|
log, err := newCLILogger(cmd)
|
|
if err != nil {
|
|
return fmt.Errorf("creating logger: %w", err)
|
|
}
|
|
defer log.Sync()
|
|
|
|
fileHandler := file.NewHandler(afero.NewOsFs())
|
|
imageFetcher := image.New()
|
|
upgrader, err := cloudcmd.NewUpgrader(cmd.OutOrStdout(), log)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return upgradeExecute(cmd, imageFetcher, upgrader, fileHandler)
|
|
}
|
|
|
|
func upgradeExecute(cmd *cobra.Command, imageFetcher imageFetcher, upgrader cloudUpgrader, fileHandler file.Handler) error {
|
|
configPath, err := cmd.Flags().GetString("config")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
conf, err := config.New(fileHandler, configPath)
|
|
if err != nil {
|
|
return displayConfigValidationErrors(cmd.ErrOrStderr(), err)
|
|
}
|
|
|
|
helm, err := cmd.Flags().GetBool("helm")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if helm {
|
|
timeout, err := cmd.Flags().GetDuration("timeout")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if err := upgrader.UpgradeHelmServices(cmd.Context(), conf, timeout); err != nil {
|
|
return fmt.Errorf("upgrading helm: %w", err)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// TODO: validate upgrade config? Should be basic things like checking image is not an empty string
|
|
// More sophisticated validation, like making sure we don't downgrade the cluster, should be done by `constellation upgrade plan`
|
|
|
|
// this config modification is temporary until we can remove the upgrade section from the config
|
|
conf.Image = conf.Upgrade.Image
|
|
imageReference, err := imageFetcher.FetchReference(cmd.Context(), conf)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return upgrader.Upgrade(cmd.Context(), imageReference, conf.Upgrade.Image, conf.Upgrade.Measurements)
|
|
}
|
|
|
|
type cloudUpgrader interface {
|
|
Upgrade(ctx context.Context, imageReference, imageVersion string, measurements measurements.M) error
|
|
UpgradeHelmServices(ctx context.Context, config *config.Config, timeout time.Duration) error
|
|
}
|
|
|
|
type imageFetcher interface {
|
|
FetchReference(ctx context.Context, config *config.Config) (string, error)
|
|
}
|