mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-23 17:55:44 +00:00
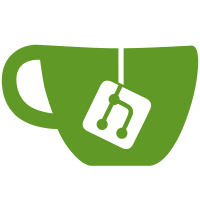
Co-authored-by: Malte Poll <mp@edgeless.systems> Co-authored-by: katexochen <katexochen@users.noreply.github.com> Co-authored-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Thomas Tendyck <tt@edgeless.systems> Co-authored-by: Benedict Schlueter <bs@edgeless.systems> Co-authored-by: leongross <leon.gross@rub.de> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com>
99 lines
2.5 KiB
Go
99 lines
2.5 KiB
Go
package client
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
computepb "google.golang.org/genproto/googleapis/cloud/compute/v1"
|
|
)
|
|
|
|
// waitForOperations waits until every operation in the opIDs slice is
|
|
// done or returns the first occurring error.
|
|
func (c *Client) waitForOperations(ctx context.Context, ops []Operation) error {
|
|
for _, op := range ops {
|
|
switch {
|
|
case op.Proto().Zone != nil:
|
|
if err := c.waitForZoneOperation(ctx, op); err != nil {
|
|
return err
|
|
}
|
|
case op.Proto().Region != nil:
|
|
if err := c.waitForRegionOperation(ctx, op); err != nil {
|
|
return err
|
|
}
|
|
default:
|
|
if err := c.waitForGlobalOperation(ctx, op); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (c *Client) waitForGlobalOperation(ctx context.Context, op Operation) error {
|
|
for {
|
|
if err := ctx.Err(); err != nil {
|
|
return err
|
|
}
|
|
waitReq := &computepb.WaitGlobalOperationRequest{
|
|
Operation: *op.Proto().Name,
|
|
Project: c.project,
|
|
}
|
|
zoneOp, err := c.operationGlobalAPI.Wait(ctx, waitReq)
|
|
if err != nil {
|
|
return fmt.Errorf("unable to wait for the operation: %w", err)
|
|
}
|
|
if *zoneOp.Status.Enum() == computepb.Operation_DONE {
|
|
if opErr := zoneOp.Error; opErr != nil {
|
|
return fmt.Errorf("operation failed: %s", opErr.String())
|
|
}
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
|
|
func (c *Client) waitForZoneOperation(ctx context.Context, op Operation) error {
|
|
for {
|
|
if err := ctx.Err(); err != nil {
|
|
return err
|
|
}
|
|
waitReq := &computepb.WaitZoneOperationRequest{
|
|
Operation: *op.Proto().Name,
|
|
Project: c.project,
|
|
Zone: c.zone,
|
|
}
|
|
zoneOp, err := c.operationZoneAPI.Wait(ctx, waitReq)
|
|
if err != nil {
|
|
return fmt.Errorf("unable to wait for the operation: %w", err)
|
|
}
|
|
if *zoneOp.Status.Enum() == computepb.Operation_DONE {
|
|
if opErr := zoneOp.Error; opErr != nil {
|
|
return fmt.Errorf("operation failed: %s", opErr.String())
|
|
}
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
|
|
func (c *Client) waitForRegionOperation(ctx context.Context, op Operation) error {
|
|
for {
|
|
if err := ctx.Err(); err != nil {
|
|
return err
|
|
}
|
|
waitReq := &computepb.WaitRegionOperationRequest{
|
|
Operation: *op.Proto().Name,
|
|
Project: c.project,
|
|
Region: c.region,
|
|
}
|
|
regionOp, err := c.operationRegionAPI.Wait(ctx, waitReq)
|
|
if err != nil {
|
|
return fmt.Errorf("unable to wait for the operation: %w", err)
|
|
}
|
|
if *regionOp.Status.Enum() == computepb.Operation_DONE {
|
|
if opErr := regionOp.Error; opErr != nil {
|
|
return fmt.Errorf("operation failed: %s", opErr.String())
|
|
}
|
|
return nil
|
|
}
|
|
}
|
|
}
|