mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
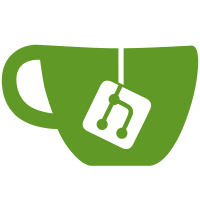
sed -i '1i/*\nCopyright (c) Edgeless Systems GmbH\n\nSPDX-License-Identifier: AGPL-3.0-only\n*/\n' `grep -rL --include='*.go' 'DO NOT EDIT'` gofumpt -w .
33 lines
780 B
Go
33 lines
780 B
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package client
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"regexp"
|
|
|
|
"google.golang.org/genproto/googleapis/cloud/compute/v1"
|
|
)
|
|
|
|
var numericProjectIDRegex = regexp.MustCompile(`^\d+$`)
|
|
|
|
// canonicalProjectID returns the project id for a given project id or project number.
|
|
func (c *Client) canonicalProjectID(ctx context.Context, project string) (string, error) {
|
|
if !numericProjectIDRegex.MatchString(project) {
|
|
return project, nil
|
|
}
|
|
computeProject, err := c.projectAPI.Get(ctx, &compute.GetProjectRequest{Project: project})
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
if computeProject == nil || computeProject.Name == nil {
|
|
return "", errors.New("invalid project")
|
|
}
|
|
return *computeProject.Name, nil
|
|
}
|