mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
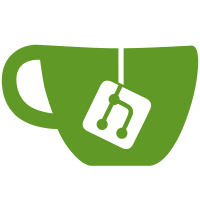
* add current chart add current helm chart * disable service controller for aws ccm * add new iam roles * doc AWS internet LB + add to LB test * pass clusterName to helm for AWS LB * fix update-aws-lb chart to also include .helmignore * move chart outside services * working state * add subnet tags for AWS subnet discovery * fix .helmignore load rule with file in subdirectory * upgrade iam profile * revert new loader impl since cilium is not correctly loaded * install chart if not already present during `upgrade apply` * cleanup PR + fix build + add todos cleanup PR + add todos * shared helm pkg for cli install and bootstrapper * add link to eks docs * refactor iamMigrationCmd * delete unused helm.symwallk * move iammigrate to upgrade pkg * fixup! delete unused helm.symwallk * add to upgradecheck * remove nodeSelector from go code (Otto) * update iam docs and sort permission + remove duplicate roles * fix bug in `upgrade check` * better upgrade check output when svc version upgrade not possible * pr feedback * remove force flag in upgrade_test * use upgrader.GetUpgradeID instead of extra type * remove todos + fix check * update doc lb (leo) * remove bootstrapper helm package * Update cli/internal/cmd/upgradecheck.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * final nits * add docs for e2e upgrade test setup * Apply suggestions from code review Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/helm/loader.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/cmd/tfmigrationclient.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * fix daniel review * link to the iam permissions instead of manually updating them (agreed with leo) * disable iam upgrade in upgrade apply --------- Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> Co-authored-by: Malte Poll
69 lines
2.5 KiB
Go
69 lines
2.5 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package cmd
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/edgelesssys/constellation/v2/cli/internal/upgrade"
|
|
"github.com/edgelesssys/constellation/v2/internal/file"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
// tfMigrationClient is a client for planning and applying Terraform migrations.
|
|
type tfMigrationClient struct {
|
|
log debugLog
|
|
}
|
|
|
|
// planMigration checks for Terraform migrations and asks for confirmation if there are any. The user input is returned as confirmedDiff.
|
|
// adapted from migrateTerraform().
|
|
func (u *tfMigrationClient) planMigration(cmd *cobra.Command, file file.Handler, migrateCmd upgrade.TfMigrationCmd) (hasDiff bool, err error) {
|
|
u.log.Debugf("Planning %s", migrateCmd.String())
|
|
if err := migrateCmd.CheckTerraformMigrations(file); err != nil {
|
|
return false, fmt.Errorf("checking workspace: %w", err)
|
|
}
|
|
hasDiff, err = migrateCmd.Plan(cmd.Context(), file, cmd.OutOrStdout())
|
|
if err != nil {
|
|
return hasDiff, fmt.Errorf("planning terraform migrations: %w", err)
|
|
}
|
|
return hasDiff, nil
|
|
}
|
|
|
|
// applyMigration plans and then applies the Terraform migration. The user is asked for confirmation if there are any changes.
|
|
// adapted from migrateTerraform().
|
|
func (u *tfMigrationClient) applyMigration(cmd *cobra.Command, file file.Handler, migrateCmd upgrade.TfMigrationCmd, flags upgradeApplyFlags) error {
|
|
hasDiff, err := u.planMigration(cmd, file, migrateCmd)
|
|
if err != nil {
|
|
return fmt.Errorf("planning terraform migrations: %w", err)
|
|
}
|
|
if hasDiff {
|
|
// If there are any Terraform migrations to apply, ask for confirmation
|
|
fmt.Fprintf(cmd.OutOrStdout(), "The %s upgrade requires a migration of Constellation cloud resources by applying an updated Terraform template. Please manually review the suggested changes below.\n", migrateCmd.String())
|
|
if !flags.yes {
|
|
ok, err := askToConfirm(cmd, fmt.Sprintf("Do you want to apply the %s?", migrateCmd.String()))
|
|
if err != nil {
|
|
return fmt.Errorf("asking for confirmation: %w", err)
|
|
}
|
|
if !ok {
|
|
cmd.Println("Aborting upgrade.")
|
|
if err := upgrade.CleanUpTerraformMigrations(migrateCmd.UpgradeID(), file); err != nil {
|
|
return fmt.Errorf("cleaning up workspace: %w", err)
|
|
}
|
|
return fmt.Errorf("aborted by user")
|
|
}
|
|
}
|
|
u.log.Debugf("Applying Terraform %s migrations", migrateCmd.String())
|
|
err := migrateCmd.Apply(cmd.Context(), file)
|
|
if err != nil {
|
|
return fmt.Errorf("applying terraform migrations: %w", err)
|
|
}
|
|
} else {
|
|
u.log.Debugf("No Terraform diff detected")
|
|
}
|
|
return nil
|
|
}
|