mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
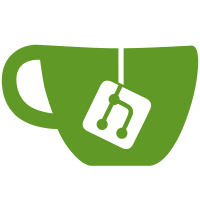
* terraform: add Azure marketplace variable Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * config: add Azure marketplace variable Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * cli: use Terraform variables from config Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * terraform: pass down marketplace variable * image: pad Azure images to 1GiB * terraform: add version attribute to marketplace image * semver: allow versions to be exported without prefix * cli: boolean var to use marketplace images * config: remove dive key * dev-docs: add instructions on how to use marketplace images * terraform: fix unit test * terraform: only fetch image for non-marketplace images * mpimage: refactor image selection Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [remove] increase minor version for image build Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * terraform: ignore changes to source_image_reference on upgrade * operator: add support for parsing Azure marketplace images Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * upgrade: fix imagefetcher call * docs: add info about azure marketplace * image: ensure more than 1GiB in size * image: test to pad to 2GiB * version: change back to v2.14.0-pre * image: GPT-conformant image size padding * [remove] increase version * mpimage: inline prefix func Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * ci: add marketplace image e2e test Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [remove] register workflow * ci: fix workflow name * ci: only allow azure test * cli: add marketplace image input to interface * cli: fix argument passing * version: roll back to v2.14.0 * ci: add force-flag support * Update docs/docs/overview/license.md * Update dev-docs/workflows/marketplace-images.md Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com> --------- Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com> Co-authored-by: Thomas Tendyck <51411342+thomasten@users.noreply.github.com>
70 lines
2.2 KiB
Go
70 lines
2.2 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
/*
|
|
imagefetch retrieves a CSP image reference from a Constellation config in the CWD.
|
|
This is especially useful when using self-managed infrastructure, where the image
|
|
reference needs to be chosen by the user, which would usually happen manually.
|
|
*/
|
|
package main
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
"regexp"
|
|
|
|
"github.com/edgelesssys/constellation/v2/internal/api/attestationconfigapi"
|
|
"github.com/edgelesssys/constellation/v2/internal/cloud/cloudprovider"
|
|
"github.com/edgelesssys/constellation/v2/internal/config"
|
|
"github.com/edgelesssys/constellation/v2/internal/constants"
|
|
"github.com/edgelesssys/constellation/v2/internal/file"
|
|
"github.com/edgelesssys/constellation/v2/internal/imagefetcher"
|
|
"github.com/spf13/afero"
|
|
)
|
|
|
|
var (
|
|
caseInsensitiveCommunityGalleriesRegexp = regexp.MustCompile(`(?i)\/communitygalleries\/`)
|
|
caseInsensitiveImagesRegExp = regexp.MustCompile(`(?i)\/images\/`)
|
|
caseInsensitiveVersionsRegExp = regexp.MustCompile(`(?i)\/versions\/`)
|
|
)
|
|
|
|
func main() {
|
|
cwd := os.Getenv("BUILD_WORKING_DIRECTORY") // set by Bazel, for bazel run compatibility
|
|
ctx := context.Background()
|
|
|
|
fh := file.NewHandler(afero.NewOsFs())
|
|
attFetcher := attestationconfigapi.NewFetcher()
|
|
conf, err := config.New(fh, filepath.Join(cwd, constants.ConfigFilename), attFetcher, true)
|
|
var configValidationErr *config.ValidationError
|
|
if errors.As(err, &configValidationErr) {
|
|
fmt.Println(configValidationErr.LongMessage())
|
|
}
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
imgFetcher := imagefetcher.New()
|
|
provider := conf.GetProvider()
|
|
attestationVariant := conf.GetAttestationConfig().GetVariant()
|
|
region := conf.GetRegion()
|
|
image, err := imgFetcher.FetchReference(ctx, provider, attestationVariant,
|
|
conf.Image, region, conf.UseMarketplaceImage())
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
if provider == cloudprovider.Azure {
|
|
image = caseInsensitiveCommunityGalleriesRegexp.ReplaceAllString(image, "/communityGalleries/")
|
|
image = caseInsensitiveImagesRegExp.ReplaceAllString(image, "/images/")
|
|
image = caseInsensitiveVersionsRegExp.ReplaceAllString(image, "/versions/")
|
|
}
|
|
|
|
fmt.Println(image)
|
|
}
|