mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
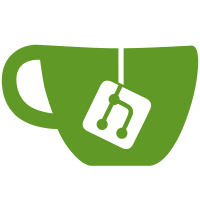
There used to be three definitions of a Component type, and conversion routines between the three. Since the use case is always the same, and the Component semantics are defined by versions.go and the installer, it seems appropriate to define the Component type there and import it in the necessary places.
177 lines
6.4 KiB
Go
177 lines
6.4 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.31.0
|
|
// protoc v4.22.1
|
|
// source: internal/versions/components/components.proto
|
|
|
|
package components
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type Component struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Url string `protobuf:"bytes,1,opt,name=url,proto3" json:"url,omitempty"`
|
|
Hash string `protobuf:"bytes,2,opt,name=hash,proto3" json:"hash,omitempty"`
|
|
InstallPath string `protobuf:"bytes,3,opt,name=install_path,json=installPath,proto3" json:"install_path,omitempty"`
|
|
Extract bool `protobuf:"varint,4,opt,name=extract,proto3" json:"extract,omitempty"`
|
|
}
|
|
|
|
func (x *Component) Reset() {
|
|
*x = Component{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_internal_versions_components_components_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Component) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Component) ProtoMessage() {}
|
|
|
|
func (x *Component) ProtoReflect() protoreflect.Message {
|
|
mi := &file_internal_versions_components_components_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Component.ProtoReflect.Descriptor instead.
|
|
func (*Component) Descriptor() ([]byte, []int) {
|
|
return file_internal_versions_components_components_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *Component) GetUrl() string {
|
|
if x != nil {
|
|
return x.Url
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Component) GetHash() string {
|
|
if x != nil {
|
|
return x.Hash
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Component) GetInstallPath() string {
|
|
if x != nil {
|
|
return x.InstallPath
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Component) GetExtract() bool {
|
|
if x != nil {
|
|
return x.Extract
|
|
}
|
|
return false
|
|
}
|
|
|
|
var File_internal_versions_components_components_proto protoreflect.FileDescriptor
|
|
|
|
var file_internal_versions_components_components_proto_rawDesc = []byte{
|
|
0x0a, 0x2d, 0x69, 0x6e, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x2f, 0x76, 0x65, 0x72, 0x73, 0x69,
|
|
0x6f, 0x6e, 0x73, 0x2f, 0x63, 0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65, 0x6e, 0x74, 0x73, 0x2f, 0x63,
|
|
0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65, 0x6e, 0x74, 0x73, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12,
|
|
0x0a, 0x63, 0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65, 0x6e, 0x74, 0x73, 0x22, 0x6e, 0x0a, 0x09, 0x43,
|
|
0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65, 0x6e, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x75, 0x72, 0x6c, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x75, 0x72, 0x6c, 0x12, 0x12, 0x0a, 0x04, 0x68, 0x61,
|
|
0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x68, 0x61, 0x73, 0x68, 0x12, 0x21,
|
|
0x0a, 0x0c, 0x69, 0x6e, 0x73, 0x74, 0x61, 0x6c, 0x6c, 0x5f, 0x70, 0x61, 0x74, 0x68, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x69, 0x6e, 0x73, 0x74, 0x61, 0x6c, 0x6c, 0x50, 0x61, 0x74,
|
|
0x68, 0x12, 0x18, 0x0a, 0x07, 0x65, 0x78, 0x74, 0x72, 0x61, 0x63, 0x74, 0x18, 0x04, 0x20, 0x01,
|
|
0x28, 0x08, 0x52, 0x07, 0x65, 0x78, 0x74, 0x72, 0x61, 0x63, 0x74, 0x42, 0x46, 0x5a, 0x44, 0x67,
|
|
0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x65, 0x64, 0x67, 0x65, 0x6c, 0x65,
|
|
0x73, 0x73, 0x73, 0x79, 0x73, 0x2f, 0x63, 0x6f, 0x6e, 0x73, 0x74, 0x65, 0x6c, 0x6c, 0x61, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x2f, 0x76, 0x32, 0x2f, 0x69, 0x6e, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x2f,
|
|
0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x73, 0x2f, 0x63, 0x6f, 0x6d, 0x70, 0x6f, 0x6e, 0x65,
|
|
0x6e, 0x74, 0x73, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_internal_versions_components_components_proto_rawDescOnce sync.Once
|
|
file_internal_versions_components_components_proto_rawDescData = file_internal_versions_components_components_proto_rawDesc
|
|
)
|
|
|
|
func file_internal_versions_components_components_proto_rawDescGZIP() []byte {
|
|
file_internal_versions_components_components_proto_rawDescOnce.Do(func() {
|
|
file_internal_versions_components_components_proto_rawDescData = protoimpl.X.CompressGZIP(file_internal_versions_components_components_proto_rawDescData)
|
|
})
|
|
return file_internal_versions_components_components_proto_rawDescData
|
|
}
|
|
|
|
var file_internal_versions_components_components_proto_msgTypes = make([]protoimpl.MessageInfo, 1)
|
|
var file_internal_versions_components_components_proto_goTypes = []interface{}{
|
|
(*Component)(nil), // 0: components.Component
|
|
}
|
|
var file_internal_versions_components_components_proto_depIdxs = []int32{
|
|
0, // [0:0] is the sub-list for method output_type
|
|
0, // [0:0] is the sub-list for method input_type
|
|
0, // [0:0] is the sub-list for extension type_name
|
|
0, // [0:0] is the sub-list for extension extendee
|
|
0, // [0:0] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_internal_versions_components_components_proto_init() }
|
|
func file_internal_versions_components_components_proto_init() {
|
|
if File_internal_versions_components_components_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_internal_versions_components_components_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Component); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_internal_versions_components_components_proto_rawDesc,
|
|
NumEnums: 0,
|
|
NumMessages: 1,
|
|
NumExtensions: 0,
|
|
NumServices: 0,
|
|
},
|
|
GoTypes: file_internal_versions_components_components_proto_goTypes,
|
|
DependencyIndexes: file_internal_versions_components_components_proto_depIdxs,
|
|
MessageInfos: file_internal_versions_components_components_proto_msgTypes,
|
|
}.Build()
|
|
File_internal_versions_components_components_proto = out.File
|
|
file_internal_versions_components_components_proto_rawDesc = nil
|
|
file_internal_versions_components_components_proto_goTypes = nil
|
|
file_internal_versions_components_components_proto_depIdxs = nil
|
|
}
|