mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-20 08:15:56 +00:00
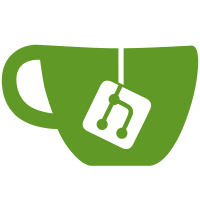
Applies the updated NodeVersion object with one request instead of two. This makes sure that the first request does not accidentially put the cluster into a "updgrade in progress" status. Which would lead users to having to run apply twice.
42 lines
1.2 KiB
Go
42 lines
1.2 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package cloudcmd
|
|
|
|
import (
|
|
"context"
|
|
"io"
|
|
|
|
"github.com/edgelesssys/constellation/v2/cli/internal/terraform"
|
|
"github.com/edgelesssys/constellation/v2/internal/cloud/cloudprovider"
|
|
"github.com/edgelesssys/constellation/v2/internal/config"
|
|
tfjson "github.com/hashicorp/terraform-json"
|
|
)
|
|
|
|
// imageFetcher gets an image reference from the versionsapi.
|
|
type imageFetcher interface {
|
|
FetchReference(ctx context.Context, config *config.Config) (string, error)
|
|
}
|
|
|
|
type terraformClient interface {
|
|
PrepareWorkspace(path string, input terraform.Variables) error
|
|
CreateCluster(ctx context.Context) (terraform.CreateOutput, error)
|
|
CreateIAMConfig(ctx context.Context, provider cloudprovider.Provider) (terraform.IAMOutput, error)
|
|
Destroy(ctx context.Context) error
|
|
CleanUpWorkspace() error
|
|
RemoveInstaller()
|
|
Show(ctx context.Context) (*tfjson.State, error)
|
|
}
|
|
|
|
type libvirtRunner interface {
|
|
Start(ctx context.Context, containerName, imageName string) error
|
|
Stop(ctx context.Context) error
|
|
}
|
|
|
|
type rawDownloader interface {
|
|
Download(ctx context.Context, errWriter io.Writer, isTTY bool, source, version string) (string, error)
|
|
}
|