mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
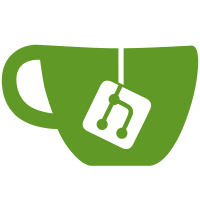
* Remove unused package * Add Go package docs to most packages Signed-off-by: Daniel Weiße <dw@edgeless.systems> Signed-off-by: Fabian Kammel <fk@edgeless.systems> Signed-off-by: Paul Meyer <49727155+katexochen@users.noreply.github.com> Co-authored-by: Paul Meyer <49727155+katexochen@users.noreply.github.com> Co-authored-by: Fabian Kammel <fk@edgeless.systems>
75 lines
2.1 KiB
Go
75 lines
2.1 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
// Package kms handles communication with Constellation's key service to request data encryption keys for new or rejoining nodes.
|
|
package kms
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/edgelesssys/constellation/v2/internal/logger"
|
|
"github.com/edgelesssys/constellation/v2/keyservice/keyserviceproto"
|
|
"go.uber.org/zap"
|
|
"google.golang.org/grpc"
|
|
"google.golang.org/grpc/credentials/insecure"
|
|
)
|
|
|
|
// Client interacts with Constellation's keyservice.
|
|
type Client struct {
|
|
log *logger.Logger
|
|
endpoint string
|
|
grpc grpcClient
|
|
}
|
|
|
|
// New creates a new KMS.
|
|
func New(log *logger.Logger, endpoint string) Client {
|
|
return Client{
|
|
log: log,
|
|
endpoint: endpoint,
|
|
grpc: client{},
|
|
}
|
|
}
|
|
|
|
// GetDataKey returns a data encryption key for the given UUID.
|
|
func (c Client) GetDataKey(ctx context.Context, keyID string, length int) ([]byte, error) {
|
|
log := c.log.With(zap.String("keyID", keyID), zap.String("endpoint", c.endpoint))
|
|
// the KMS does not use aTLS since traffic is only routed through the Constellation cluster
|
|
// cluster internal connections are considered trustworthy
|
|
log.Infof("Connecting to KMS at %s", c.endpoint)
|
|
conn, err := grpc.DialContext(ctx, c.endpoint, grpc.WithTransportCredentials(insecure.NewCredentials()))
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
defer conn.Close()
|
|
|
|
log.Infof("Requesting data key")
|
|
res, err := c.grpc.GetDataKey(
|
|
ctx,
|
|
&keyserviceproto.GetDataKeyRequest{
|
|
DataKeyId: keyID,
|
|
Length: uint32(length),
|
|
},
|
|
conn,
|
|
)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("fetching data encryption key from Constellation KMS: %w", err)
|
|
}
|
|
|
|
log.Infof("Data key request successful")
|
|
return res.DataKey, nil
|
|
}
|
|
|
|
type grpcClient interface {
|
|
GetDataKey(context.Context, *keyserviceproto.GetDataKeyRequest, *grpc.ClientConn) (*keyserviceproto.GetDataKeyResponse, error)
|
|
}
|
|
|
|
type client struct{}
|
|
|
|
func (c client) GetDataKey(ctx context.Context, req *keyserviceproto.GetDataKeyRequest, conn *grpc.ClientConn) (*keyserviceproto.GetDataKeyResponse, error) {
|
|
return keyserviceproto.NewAPIClient(conn).GetDataKey(ctx, req)
|
|
}
|