mirror of
https://github.com/edgelesssys/constellation.git
synced 2025-01-22 05:11:23 -05:00
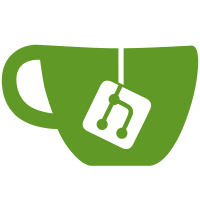
GitHub seemingly now adds a newline to the output of the gh CLI, so we need to cut it before using it.
38 lines
1.2 KiB
Bash
Executable File
38 lines
1.2 KiB
Bash
Executable File
#!/usr/bin/env bash
|
|
|
|
# get_artifact_id retrieves the artifact id of
|
|
# an artifact that was generated by a workflow.
|
|
# $1 should be the workflow run id. $2 should be the artifact name.
|
|
function get_artifact_id {
|
|
artifact_id="$(gh api \
|
|
-H "Accept: application/vnd.github+json" \
|
|
-H "X-GitHub-Api-Version: 2022-11-28" \
|
|
--paginate \
|
|
"/repos/edgelesssys/constellation/actions/runs/$1/artifacts" --jq ".artifacts |= map(select(.name==\"$2\")) | .artifacts[0].id" || exit 1)"
|
|
echo "$artifact_id" | tr -d "\n"
|
|
}
|
|
|
|
# delete_artifact_by_id deletes an artifact by its artifact id.
|
|
# $1 should be the id of the artifact.
|
|
function delete_artifact_by_id {
|
|
gh api \
|
|
--method DELETE \
|
|
-H "Accept: application/vnd.github+json" \
|
|
-H "X-GitHub-Api-Version: 2022-11-28" \
|
|
"/repos/edgelesssys/constellation/actions/artifacts/$1" || exit 1
|
|
}
|
|
|
|
workflow_id="$1"
|
|
artifact_name="$2"
|
|
|
|
if [[ -z $workflow_id ]] || [[ -z $artifact_name ]]; then
|
|
echo "Usage: delete_artifact.sh <WORKFLOW_ID> <ARTIFACT_NAME>"
|
|
exit 1
|
|
fi
|
|
|
|
echo "[*] retrieving artifact ID"
|
|
artifact_id="$(get_artifact_id "$workflow_id" "$artifact_name")"
|
|
|
|
echo "[*] deleting artifact with ID $artifact_id"
|
|
delete_artifact_by_id "$artifact_id"
|