mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-19 15:56:03 +00:00
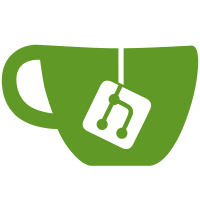
* add Azure Terraform module * add maa-patching command to cli * refactor release process * factor out image fetching to own action * add CI * generate * fix some unnecessary changes Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * use `constellation maa-patch` in ci * insecure flag when using debug image Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * only update maa url if existing Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * make node group zone optional on aws and gcp Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * [remove] register updated workflow Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * Revert "[remove] register updated workflow" This reverts commit e70b9515b7eabbcbe0d41fa1296c48750cd02ace. * create MAA Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * make maa-patching only run on azure Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * add comment Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * require node group zone for GCP and AWS * remove unnecessary bazel action * stamp version to correct file * refer to `maa-patch` command in docs * run Azure test in weekly e2e * comment / naming improvements * remove sa_account resource * disable spellcheck ot use "URL" * `create_maa` variable * don't write maa url to config Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * default to nightly image * use input ref and stream * fix command check * don't set region in weekly e2e call * patch maa if url is not empty Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * remove `create_maa` variable * remove binaries Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * remove undefined input * replace invalid attestation URL error message Co-authored-by: Thomas Tendyck <51411342+thomasten@users.noreply.github.com> * fix punctuation Co-authored-by: Thomas Tendyck <51411342+thomasten@users.noreply.github.com> * skip hidden commands in clidocgen Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * enable spellcheck before code block * move spellcheck trigger out of info block Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> * fix workflow dependencies * let image default to CLI version --------- Signed-off-by: Moritz Sanft <58110325+msanft@users.noreply.github.com> Co-authored-by: Thomas Tendyck <51411342+thomasten@users.noreply.github.com>
70 lines
1.8 KiB
Go
70 lines
1.8 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
// Clidocgen generates a Markdown page describing all CLI commands.
|
|
package main
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
"regexp"
|
|
|
|
"github.com/edgelesssys/constellation/v2/cli/cmd"
|
|
"github.com/spf13/cobra"
|
|
"github.com/spf13/cobra/doc"
|
|
)
|
|
|
|
var seeAlsoRegexp = regexp.MustCompile(`(?s)### SEE ALSO\n.+?\n\n`)
|
|
|
|
func main() {
|
|
cobra.EnableCommandSorting = false
|
|
rootCmd := cmd.NewRootCmd()
|
|
rootCmd.DisableAutoGenTag = true
|
|
|
|
// Generate Markdown for all commands.
|
|
cmdList := &bytes.Buffer{}
|
|
body := &bytes.Buffer{}
|
|
for _, c := range allSubCommands(rootCmd) {
|
|
if c.Hidden {
|
|
continue
|
|
}
|
|
name := c.Name()
|
|
fullName, level := determineFullNameAndLevel(c)
|
|
|
|
// First two arguments are used to create indentation for nested commands (2 spaces per level).
|
|
fmt.Fprintf(cmdList, "%*s* [%v](#constellation-%v): %v\n", 2*level, "", name, fullName, c.Short)
|
|
if err := doc.GenMarkdown(c, body); err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
// Remove "see also" sections. They list parent and child commands, which is not interesting for us.
|
|
cleanedBody := seeAlsoRegexp.ReplaceAll(body.Bytes(), nil)
|
|
|
|
fmt.Printf("Commands:\n\n%s\n%s", cmdList, cleanedBody)
|
|
}
|
|
|
|
func allSubCommands(cmd *cobra.Command) []*cobra.Command {
|
|
var all []*cobra.Command
|
|
for _, c := range cmd.Commands() {
|
|
all = append(all, c)
|
|
all = append(all, allSubCommands(c)...)
|
|
}
|
|
return all
|
|
}
|
|
|
|
func determineFullNameAndLevel(cmd *cobra.Command) (string, int) {
|
|
// Traverse the command tree upwards and determine the full name and level of the command.
|
|
name := cmd.Name()
|
|
level := 0
|
|
for cmd.HasParent() && cmd.Parent().Name() != "constellation" {
|
|
cmd = cmd.Parent()
|
|
name = cmd.Name() + "-" + name // Use '-' as separator since we pipe it into a Markdown link.
|
|
level++
|
|
}
|
|
return name, level
|
|
}
|