mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
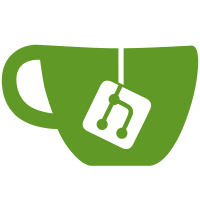
* config: disable user-facing version fetch for Azure SEV SNP don't allow "latest" value and disable user-facing version fetcher for Azure SEV SNP Co-authored-by: @derpsteb * fix unittests * attestation: getTrustedKey --------- Co-authored-by: Otto Bittner <cobittner@posteo.net>
87 lines
2.3 KiB
Go
87 lines
2.3 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package config
|
|
|
|
import (
|
|
"encoding/json"
|
|
"errors"
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
const placeholderVersionValue = 0
|
|
|
|
// NewLatestPlaceholderVersion returns the latest version with a placeholder version value.
|
|
func NewLatestPlaceholderVersion() AttestationVersion {
|
|
return AttestationVersion{
|
|
Value: placeholderVersionValue,
|
|
IsLatest: true,
|
|
}
|
|
}
|
|
|
|
// AttestationVersion is a type that represents a version of a SNP.
|
|
type AttestationVersion struct {
|
|
Value uint8
|
|
IsLatest bool
|
|
}
|
|
|
|
// MarshalYAML implements a custom marshaller to resolve "latest" values.
|
|
func (v AttestationVersion) MarshalYAML() (any, error) {
|
|
if v.IsLatest {
|
|
return "latest", nil
|
|
}
|
|
return int(v.Value), nil
|
|
}
|
|
|
|
// UnmarshalYAML implements a custom unmarshaller to resolve "atest" values.
|
|
func (v *AttestationVersion) UnmarshalYAML(unmarshal func(any) error) error {
|
|
var rawUnmarshal any
|
|
if err := unmarshal(&rawUnmarshal); err != nil {
|
|
return fmt.Errorf("raw unmarshal: %w", err)
|
|
}
|
|
|
|
return v.parseRawUnmarshal(rawUnmarshal)
|
|
}
|
|
|
|
// MarshalJSON implements a custom marshaller to resolve "latest" values.
|
|
func (v AttestationVersion) MarshalJSON() ([]byte, error) {
|
|
if v.IsLatest {
|
|
return json.Marshal("latest")
|
|
}
|
|
return json.Marshal(v.Value)
|
|
}
|
|
|
|
// UnmarshalJSON implements a custom unmarshaller to resolve "latest" values.
|
|
func (v *AttestationVersion) UnmarshalJSON(data []byte) (err error) {
|
|
var rawUnmarshal any
|
|
if err := json.Unmarshal(data, &rawUnmarshal); err != nil {
|
|
return fmt.Errorf("raw unmarshal: %w", err)
|
|
}
|
|
return v.parseRawUnmarshal(rawUnmarshal)
|
|
}
|
|
|
|
func (v *AttestationVersion) parseRawUnmarshal(rawUnmarshal any) error {
|
|
switch s := rawUnmarshal.(type) {
|
|
case string:
|
|
if strings.ToLower(s) == "latest" {
|
|
// TODO(elchead): activate latest logic for next release AB#3036
|
|
return errors.New("latest is not supported as a version value")
|
|
// v.IsLatest = true
|
|
// v.Value = placeholderVersionValue
|
|
}
|
|
return fmt.Errorf("invalid version value: %s", s)
|
|
case int:
|
|
v.Value = uint8(s)
|
|
// yaml spec allows "1" as float64, so version number might come as a float: https://github.com/go-yaml/yaml/issues/430
|
|
case float64:
|
|
v.Value = uint8(s)
|
|
default:
|
|
return fmt.Errorf("invalid version value type: %s", s)
|
|
}
|
|
return nil
|
|
}
|