mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-23 09:46:05 +00:00
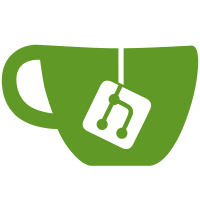
Co-authored-by: Malte Poll <mp@edgeless.systems> Co-authored-by: katexochen <katexochen@users.noreply.github.com> Co-authored-by: Daniel Weiße <dw@edgeless.systems> Co-authored-by: Thomas Tendyck <tt@edgeless.systems> Co-authored-by: Benedict Schlueter <bs@edgeless.systems> Co-authored-by: leongross <leon.gross@rub.de> Co-authored-by: Moritz Eckert <m1gh7ym0@gmail.com>
44 lines
865 B
Go
44 lines
865 B
Go
//go:build aws
|
|
// +build aws
|
|
|
|
package aws
|
|
|
|
// #include <nsm.h>
|
|
import "C"
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
// As defined by the attestation document's COSE_Sign1 structure
|
|
const nsmMaxAttestationDocSize = 16 * 1024
|
|
|
|
func NsmGetAttestationDoc(userData []byte, nonce []byte) ([]byte, error) {
|
|
doc := make([]byte, nsmMaxAttestationDocSize)
|
|
doclen := C.uint32_t(len(doc))
|
|
|
|
nsm_fd := C.nsm_lib_init()
|
|
if nsm_fd < 0 {
|
|
return nil, fmt.Errorf("could not open NSM module")
|
|
}
|
|
defer C.nsm_lib_exit(nsm_fd)
|
|
|
|
errCode := C.nsm_get_attestation_doc(
|
|
nsm_fd,
|
|
(*C.uint8_t)(&userData[0]),
|
|
C.uint32_t(len(userData)),
|
|
(*C.uint8_t)(&nonce[0]),
|
|
C.uint32_t(len(nonce)),
|
|
nil,
|
|
0,
|
|
(*C.uint8_t)(&doc[0]),
|
|
&doclen,
|
|
)
|
|
if errCode != C.ERROR_CODE_SUCCESS {
|
|
return nil, fmt.Errorf("failed to generate attestation document: %d", errCode)
|
|
}
|
|
doc = doc[:doclen]
|
|
|
|
return doc, nil
|
|
}
|