mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-12-27 00:19:36 -05:00
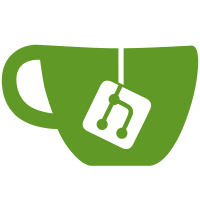
* add current chart add current helm chart * disable service controller for aws ccm * add new iam roles * doc AWS internet LB + add to LB test * pass clusterName to helm for AWS LB * fix update-aws-lb chart to also include .helmignore * move chart outside services * working state * add subnet tags for AWS subnet discovery * fix .helmignore load rule with file in subdirectory * upgrade iam profile * revert new loader impl since cilium is not correctly loaded * install chart if not already present during `upgrade apply` * cleanup PR + fix build + add todos cleanup PR + add todos * shared helm pkg for cli install and bootstrapper * add link to eks docs * refactor iamMigrationCmd * delete unused helm.symwallk * move iammigrate to upgrade pkg * fixup! delete unused helm.symwallk * add to upgradecheck * remove nodeSelector from go code (Otto) * update iam docs and sort permission + remove duplicate roles * fix bug in `upgrade check` * better upgrade check output when svc version upgrade not possible * pr feedback * remove force flag in upgrade_test * use upgrader.GetUpgradeID instead of extra type * remove todos + fix check * update doc lb (leo) * remove bootstrapper helm package * Update cli/internal/cmd/upgradecheck.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * final nits * add docs for e2e upgrade test setup * Apply suggestions from code review Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/helm/loader.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * Update cli/internal/cmd/tfmigrationclient.go Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> * fix daniel review * link to the iam permissions instead of manually updating them (agreed with leo) * disable iam upgrade in upgrade apply --------- Co-authored-by: Daniel Weiße <66256922+daniel-weisse@users.noreply.github.com> Co-authored-by: Malte Poll
62 lines
1.8 KiB
Go
62 lines
1.8 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
// Package helm provides types and functions shared across services.
|
|
package helm
|
|
|
|
// Release bundles all information necessary to create a helm release.
|
|
type Release struct {
|
|
Chart []byte
|
|
Values map[string]any
|
|
ReleaseName string
|
|
WaitMode WaitMode
|
|
}
|
|
|
|
// Releases bundles all helm releases to be deployed to Constellation.
|
|
type Releases struct {
|
|
AWSLoadBalancerController *Release
|
|
CSI *Release
|
|
Cilium Release
|
|
CertManager Release
|
|
ConstellationOperators Release
|
|
ConstellationServices Release
|
|
}
|
|
|
|
// MergeMaps returns a new map that is the merger of it's inputs.
|
|
// Key collisions are resolved by taking the value of the second argument (map b).
|
|
// Taken from: https://github.com/helm/helm/blob/dbc6d8e20fe1d58d50e6ed30f09a04a77e4c68db/pkg/cli/values/options.go#L91-L108.
|
|
func MergeMaps(a, b map[string]any) map[string]any {
|
|
out := make(map[string]any, len(a))
|
|
for k, v := range a {
|
|
out[k] = v
|
|
}
|
|
for k, v := range b {
|
|
if v, ok := v.(map[string]any); ok {
|
|
if bv, ok := out[k]; ok {
|
|
if bv, ok := bv.(map[string]any); ok {
|
|
out[k] = MergeMaps(bv, v)
|
|
continue
|
|
}
|
|
}
|
|
}
|
|
out[k] = v
|
|
}
|
|
return out
|
|
}
|
|
|
|
// WaitMode specifies the wait mode for a helm release.
|
|
type WaitMode string
|
|
|
|
const (
|
|
// WaitModeNone specifies that the helm release should not wait for the resources to be ready.
|
|
WaitModeNone WaitMode = ""
|
|
// WaitModeWait specifies that the helm release should wait for the resources to be ready.
|
|
WaitModeWait WaitMode = "wait"
|
|
// WaitModeAtomic specifies that the helm release should
|
|
// wait for the resources to be ready and roll back atomically on failure.
|
|
WaitModeAtomic WaitMode = "atomic"
|
|
)
|