mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
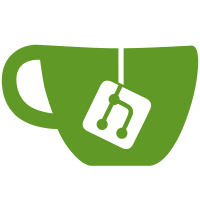
* build: correct toolchain order * build: gazelle-update-repos * build: use pregenerated proto for dependencies * update bazeldnf * deps: tpm simulator * Update Google trillian module * cli: add stamping as alternative build info source * bazel: add go_test wrappers, mark special tests and select testing deps * deps: add libvirt deps * deps: go-libvirt patches * deps: cloudflare circl patches * bazel: add go_test wrappers, mark special tests and select testing deps * bazel: keep gazelle overrides * bazel: cleanup bazelrc * bazel: switch CMakeLists.txt to use bazel * bazel: fix injection of version information via stamping * bazel: commit all build files * dev-docs: document bazel usage * deps: upgrade zig-cc for go 1.20 * bazel: update Perl for macOS arm64 & Linux arm64 support * bazel: use static perl toolchain for OpenSSL * bazel: use static protobuf (protoc) toolchain * deps: add git and go to nix deps Co-authored-by: Paul Meyer <49727155+katexochen@users.noreply.github.com>
71 lines
1.5 KiB
Go
71 lines
1.5 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package cmd
|
|
|
|
import (
|
|
"bytes"
|
|
"errors"
|
|
"io"
|
|
"testing"
|
|
|
|
"github.com/spf13/cobra"
|
|
"github.com/stretchr/testify/assert"
|
|
)
|
|
|
|
func TestAskToConfirm(t *testing.T) {
|
|
// errAborted is an error where the user aborted the action.
|
|
errAborted := errors.New("user aborted")
|
|
|
|
cmd := &cobra.Command{
|
|
Use: "test",
|
|
Args: cobra.NoArgs,
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
ok, err := askToConfirm(cmd, "777")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if !ok {
|
|
return errAborted
|
|
}
|
|
return nil
|
|
},
|
|
}
|
|
|
|
testCases := map[string]struct {
|
|
input string
|
|
wantErr error
|
|
}{
|
|
"user confirms": {"y\n", nil},
|
|
"user confirms long": {"yes\n", nil},
|
|
"user disagrees": {"n\n", errAborted},
|
|
"user disagrees long": {"no\n", errAborted},
|
|
"user is first unsure, but agrees": {"what?\ny\n", nil},
|
|
"user is first unsure, but disagrees": {"wait.\nn\n", errAborted},
|
|
"repeated invalid input": {"h\nb\nq\n", ErrInvalidInput},
|
|
}
|
|
|
|
for name, tc := range testCases {
|
|
t.Run(name, func(t *testing.T) {
|
|
assert := assert.New(t)
|
|
|
|
out := &bytes.Buffer{}
|
|
cmd.SetOut(out)
|
|
cmd.SetErr(&bytes.Buffer{})
|
|
in := bytes.NewBufferString(tc.input)
|
|
cmd.SetIn(in)
|
|
cmd.SetArgs([]string{})
|
|
|
|
err := cmd.Execute()
|
|
assert.ErrorIs(err, tc.wantErr)
|
|
|
|
output, err := io.ReadAll(out)
|
|
assert.NoError(err)
|
|
assert.Contains(string(output), "777")
|
|
})
|
|
}
|
|
}
|