mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-10-01 01:36:09 -04:00
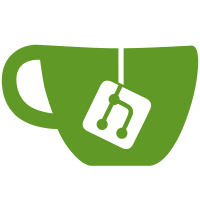
sed -i '1i/*\nCopyright (c) Edgeless Systems GmbH\n\nSPDX-License-Identifier: AGPL-3.0-only\n*/\n' `grep -rL --include='*.go' 'DO NOT EDIT'` gofumpt -w .
40 lines
1.0 KiB
Go
40 lines
1.0 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package client
|
|
|
|
import (
|
|
"encoding/json"
|
|
"errors"
|
|
|
|
"github.com/spf13/afero"
|
|
)
|
|
|
|
// cloudConfig uses same format as azure cloud controller manager to share the same kubernetes secret.
|
|
// this definition only contains the fields we need.
|
|
// reference: https://cloud-provider-azure.sigs.k8s.io/install/configs/ .
|
|
type cloudConfig struct {
|
|
TenantID string `json:"tenantId,omitempty"`
|
|
SubscriptionID string `json:"subscriptionId,omitempty"`
|
|
ResourceGroup string `json:"resourceGroup,omitempty"`
|
|
}
|
|
|
|
// loadConfig loads the cloud config from the given path.
|
|
func loadConfig(fs afero.Fs, path string) (*cloudConfig, error) {
|
|
rawConfig, err := afero.ReadFile(fs, path)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
var config cloudConfig
|
|
if err := json.Unmarshal(rawConfig, &config); err != nil {
|
|
return nil, err
|
|
}
|
|
if config.TenantID == "" || config.SubscriptionID == "" {
|
|
return nil, errors.New("invalid config: tenantId and subscriptionId are required")
|
|
}
|
|
return &config, nil
|
|
}
|