mirror of
https://github.com/edgelesssys/constellation.git
synced 2024-09-20 08:15:56 +00:00
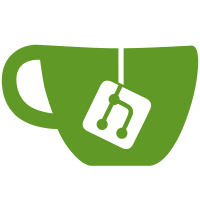
* Include EXC0014 and fix issues. * Include EXC0012 and fix issues. Signed-off-by: Fabian Kammel <fk@edgeless.systems> Co-authored-by: Otto Bittner <cobittner@posteo.net>
57 lines
1.5 KiB
Go
57 lines
1.5 KiB
Go
/*
|
|
Copyright (c) Edgeless Systems GmbH
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
*/
|
|
|
|
package azure
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"fmt"
|
|
|
|
"github.com/microsoft/ApplicationInsights-Go/appinsights"
|
|
)
|
|
|
|
// Logger implements CloudLogger interface for Azure to Disclose early boot
|
|
// logs into Azure's App Insights service.
|
|
type Logger struct {
|
|
client appinsights.TelemetryClient
|
|
}
|
|
|
|
// NewLogger creates a new client to store information in Azure Application Insights
|
|
// https://github.com/Microsoft/ApplicationInsights-go
|
|
func NewLogger(ctx context.Context, metadata *Metadata) (*Logger, error) {
|
|
component, err := metadata.getAppInsights(ctx)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("getting app insights: %w", err)
|
|
}
|
|
|
|
if component.Properties == nil || component.Properties.InstrumentationKey == nil {
|
|
return nil, errors.New("unable to get instrumentation key")
|
|
}
|
|
|
|
client := appinsights.NewTelemetryClient(*component.Properties.InstrumentationKey)
|
|
|
|
self, err := metadata.Self(ctx)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("getting self: %w", err)
|
|
}
|
|
client.Context().CommonProperties["instance-name"] = self.Name
|
|
|
|
return &Logger{client: client}, nil
|
|
}
|
|
|
|
// Disclose stores log information in Azure Application Insights!
|
|
// Do **NOT** log sensitive information!
|
|
func (l *Logger) Disclose(msg string) {
|
|
l.client.Track(appinsights.NewTraceTelemetry(msg, appinsights.Information))
|
|
}
|
|
|
|
// Close blocks until all information are written to cloud API.
|
|
func (l *Logger) Close() error {
|
|
<-l.client.Channel().Close()
|
|
return nil
|
|
}
|