mirror of
https://github.com/srlabs/blue-merle.git
synced 2025-01-10 14:49:35 -05:00
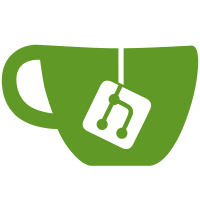
I have rsynced the whole device before associating with a new device and after. The only file that got modified was /etc/oui-tertf/client.db. We intend to have it stored in memory rather than on flash. This should be okay since the kernel also holds the MAC addresses in memory.
72 lines
2.1 KiB
Bash
72 lines
2.1 KiB
Bash
#!/usr/bin/env ash
|
|
|
|
# This script provides helper functions for blue-merle
|
|
|
|
|
|
UNICAST_MAC_GEN () {
|
|
loc_mac_numgen=`python3 -c "import random; print(f'{random.randint(0,2**48) & 0b111111101111111111111111111111111111111111111111:0x}'.zfill(12))"`
|
|
loc_mac_formatted=$(echo "$loc_mac_numgen" | sed 's/^\(..\)\(..\)\(..\)\(..\)\(..\)\(..\).*$/\1:\2:\3:\4:\5:\6/')
|
|
echo "$loc_mac_formatted"
|
|
}
|
|
|
|
# randomize BSSID
|
|
RESET_BSSIDS () {
|
|
uci set wireless.@wifi-iface[1].macaddr=`UNICAST_MAC_GEN`
|
|
uci set wireless.@wifi-iface[0].macaddr=`UNICAST_MAC_GEN`
|
|
uci commit wireless
|
|
wifi # need to reset wifi for changes to apply
|
|
}
|
|
|
|
READ_IMEI () {
|
|
local answer=1
|
|
while [[ "$answer" -eq 1 ]]; do
|
|
local imei=$(gl_modem AT AT+GSN | grep -w -E "[0-9]{14,15}")
|
|
if [[ $? -eq 1 ]]; then
|
|
echo -n "Failed to read IMEI. Try again? (Y/n): "
|
|
read answer
|
|
case $answer in
|
|
n*) answer=0;;
|
|
N*) answer=0;;
|
|
*) answer=1;;
|
|
esac
|
|
if [[ $answer -eq 0 ]]; then
|
|
exit 1
|
|
fi
|
|
else
|
|
answer=0
|
|
fi
|
|
done
|
|
echo $imei
|
|
}
|
|
|
|
READ_IMSI () {
|
|
local answer=1
|
|
while [[ "$answer" -eq 1 ]]; do
|
|
local imsi=$(gl_modem AT AT+CIMI | grep -w -E "[0-9]{6,15}")
|
|
if [[ $? -eq 1 ]]; then
|
|
echo -n "Failed to read IMSI. Try again? (Y/n): "
|
|
read answer
|
|
case $answer in
|
|
n*) answer=0;;
|
|
N*) answer=0;;
|
|
*) answer=1;;
|
|
esac
|
|
if [[ $answer -eq 0 ]]; then
|
|
exit 1
|
|
fi
|
|
else
|
|
answer=0
|
|
fi
|
|
done
|
|
echo $imsi
|
|
}
|
|
|
|
CHECK_ABORT () {
|
|
sim_change_switch=`cat /tmp/sim_change_switch`
|
|
if [[ "$sim_change_switch" = "off" ]]; then
|
|
e750-mcu "SIM change aborted."
|
|
sleep 1
|
|
exit 1
|
|
fi
|
|
}
|