mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-02-27 18:31:25 -05:00
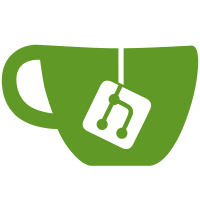
Validated chunks are shared to other peers. Force check is now very simple since it just turns all chunks into "needs checking" mode and sums are asked to sources. Sources maintain a temporary cache of chunks. Since sums are requested sparsely, this should not affect the sources in terms of performance. We can still imagine precomputing and saving sha1 of chunks while hashing them. For backward compatibility reasons, the following has been setup *temporarily* in this version: - unvalidated chunks are still considered as already obtained, and are shared and saved - force check has been disabled - final file check is maintained - in case of file fail, the old checking mode will be used. All changes for next version are kept in the define 'USE_NEW_CHUNK_CHECKING_CODE' that will be made the default in a few weeks. At start, I expect most chunk to stya yellow during download, until most sources are able to provide chunk hashs. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@5019 b45a01b8-16f6-495d-af2f-9b41ad6348cc
193 lines
6.8 KiB
C++
193 lines
6.8 KiB
C++
/*
|
|
* libretroshare/src/ft: ftdata.h
|
|
*
|
|
* File Transfer for RetroShare.
|
|
*
|
|
* Copyright 2008 by Robert Fernie.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "retroshare@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
#ifndef FT_DATA_INTERFACE_HEADER
|
|
#define FT_DATA_INTERFACE_HEADER
|
|
|
|
/*
|
|
* ftData.
|
|
*
|
|
* Internal Interfaces for sending and receiving data.
|
|
* Most likely to be implemented by ftServer.
|
|
* Provided as an independent interface for testing purposes.
|
|
*
|
|
*/
|
|
|
|
#include <string>
|
|
#include <inttypes.h>
|
|
|
|
#include <retroshare/rstypes.h>
|
|
|
|
/*************** SEND INTERFACE *******************/
|
|
|
|
class CompressedChunkMap ;
|
|
class Sha1CheckSum ;
|
|
|
|
class ftDataSend
|
|
{
|
|
public:
|
|
virtual ~ftDataSend() { return; }
|
|
|
|
/* Client Send */
|
|
virtual bool sendDataRequest(const std::string& peerId, const std::string& hash, uint64_t size, uint64_t offset, uint32_t chunksize) = 0;
|
|
|
|
/* Server Send */
|
|
virtual bool sendData(const std::string& peerId, const std::string& hash, uint64_t size, uint64_t offset, uint32_t chunksize, void *data) = 0;
|
|
|
|
/// Send a chunkmap[request]. Because requests/chunkmaps can go both
|
|
//directions, but for different usages, we have this "is_client" flags,
|
|
//that gives the ultimate goal of the data. "is_client==true" means that
|
|
//the message is for a client (download) instead of a server.
|
|
//
|
|
virtual bool sendChunkMapRequest(const std::string& peer_id,const std::string& hash,bool is_client) = 0;
|
|
virtual bool sendChunkMap(const std::string& peer_id,const std::string& hash,const CompressedChunkMap& cmap,bool is_client) = 0;
|
|
|
|
/// Send a request for a chunk crc map
|
|
virtual bool sendCRC32MapRequest(const std::string& peer_id,const std::string& hash) = 0;
|
|
/// Send a chunk crc map
|
|
virtual bool sendCRC32Map(const std::string& peer_id,const std::string& hash,const CRC32Map& crc_map) = 0;
|
|
/// Send a request for a chunk crc map
|
|
virtual bool sendSingleChunkCRCRequest(const std::string& peer_id,const std::string& hash,uint32_t chunk_number) = 0;
|
|
/// Send a chunk crc map
|
|
virtual bool sendSingleChunkCRC(const std::string& peer_id,const std::string& hash,uint32_t chunk_number,const Sha1CheckSum& crc) = 0;
|
|
};
|
|
|
|
|
|
|
|
/*************** RECV INTERFACE *******************/
|
|
|
|
class ftDataRecv
|
|
{
|
|
public:
|
|
virtual ~ftDataRecv() { return; }
|
|
|
|
/* Client Recv */
|
|
virtual bool recvData(const std::string& peerId, const std::string& hash, uint64_t size, uint64_t offset, uint32_t chunksize, void *data) = 0;
|
|
|
|
/* Server Recv */
|
|
virtual bool recvDataRequest(const std::string& peerId, const std::string& hash, uint64_t size, uint64_t offset, uint32_t chunksize) = 0;
|
|
|
|
/// Send a request for a chunk map
|
|
virtual bool recvChunkMapRequest(const std::string& peer_id,const std::string& hash,bool is_client) = 0;
|
|
|
|
/// Send a chunk map
|
|
virtual bool recvChunkMap(const std::string& peer_id,const std::string& hash,const CompressedChunkMap& cmap,bool is_client) = 0;
|
|
/// Send a request for a chunk map
|
|
virtual bool recvCRC32MapRequest(const std::string& peer_id,const std::string& hash) = 0;
|
|
|
|
/// Send a chunk map
|
|
virtual bool recvCRC32Map(const std::string& peer_id,const std::string& hash,const CRC32Map& crcmap) = 0;
|
|
};
|
|
|
|
/**************** FOR TESTING ***********************/
|
|
|
|
/******* Pair of Send/Recv (Only need to handle Send side) ******/
|
|
class ftDataSendPair: public ftDataSend
|
|
{
|
|
public:
|
|
|
|
ftDataSendPair(ftDataRecv *recv);
|
|
virtual ~ftDataSendPair() { return; }
|
|
|
|
/* Client Send */
|
|
virtual bool sendDataRequest(const std::string &peerId, const std::string &hash,
|
|
uint64_t size, uint64_t offset, uint32_t chunksize);
|
|
|
|
/* Server Send */
|
|
virtual bool sendData(const std::string &peerId, const std::string &hash, uint64_t size,
|
|
uint64_t offset, uint32_t chunksize, void *data);
|
|
|
|
/* Send a request for a chunk map */
|
|
virtual bool sendChunkMapRequest(const std::string& peer_id,const std::string& hash,bool is_client);
|
|
|
|
/* Send a chunk map */
|
|
virtual bool sendChunkMap(const std::string& peer_id,const std::string& hash, const CompressedChunkMap& cmap,bool is_client);
|
|
|
|
/* Send a request for a chunk map */
|
|
virtual bool sendCRC32MapRequest(const std::string& peer_id,const std::string& hash);
|
|
|
|
/* Send a chunk map */
|
|
virtual bool sendCRC32Map(const std::string& peer_id,const std::string& hash, const CRC32Map& cmap);
|
|
ftDataRecv *mDataRecv;
|
|
};
|
|
|
|
|
|
class ftDataSendDummy: public ftDataSend
|
|
{
|
|
public:
|
|
virtual ~ftDataSendDummy() { return; }
|
|
|
|
/* Client Send */
|
|
virtual bool sendDataRequest(const std::string &peerId, const std::string &hash,
|
|
uint64_t size, uint64_t offset, uint32_t chunksize);
|
|
|
|
/* Server Send */
|
|
virtual bool sendData(const std::string &peerId, const std::string &hash, uint64_t size,
|
|
uint64_t offset, uint32_t chunksize, void *data);
|
|
|
|
/* Send a request for a chunk map */
|
|
virtual bool sendChunkMapRequest(const std::string& peer_id,const std::string& hash,bool is_client);
|
|
|
|
/* Send a chunk map */
|
|
virtual bool sendChunkMap(const std::string& peer_id,const std::string& hash, const CompressedChunkMap& cmap,bool is_client);
|
|
|
|
/* Send a request for a chunk map */
|
|
virtual bool sendCRC32MapRequest(const std::string& peer_id,const std::string& hash);
|
|
|
|
/* Send a chunk map */
|
|
virtual bool sendCRC32Map(const std::string& peer_id,const std::string& hash, const CRC32Map& cmap);
|
|
};
|
|
|
|
class ftDataRecvDummy: public ftDataRecv
|
|
{
|
|
public:
|
|
|
|
virtual ~ftDataRecvDummy() { return; }
|
|
|
|
/* Client Recv */
|
|
virtual bool recvData(const std::string& peerId, const std::string& hash,
|
|
uint64_t size, uint64_t offset, uint32_t chunksize, void *data);
|
|
|
|
/* Server Recv */
|
|
virtual bool recvDataRequest(const std::string& peerId, const std::string& hash,
|
|
uint64_t size, uint64_t offset, uint32_t chunksize);
|
|
|
|
/* Send a request for a chunk map */
|
|
virtual bool recvChunkMapRequest(const std::string& peer_id,const std::string& hash,
|
|
bool is_client);
|
|
|
|
/* Send a chunk map */
|
|
virtual bool recvChunkMap(const std::string& peer_id,const std::string& hash,
|
|
const CompressedChunkMap& cmap,bool is_client);
|
|
|
|
/* Send a request for a chunk map */
|
|
virtual bool sendCRC32MapRequest(const std::string& peer_id,const std::string& hash);
|
|
|
|
/* Send a chunk map */
|
|
virtual bool sendCRC32Map(const std::string& peer_id,const std::string& hash, const CompressedChunkMap& cmap);
|
|
};
|
|
|
|
#endif
|