mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-07-15 10:49:32 -04:00
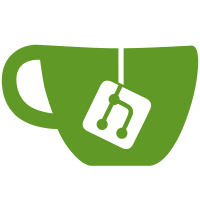
* Added GUI interface for auto connect state. * Added HTTP retrieval and storage of DHT peers update. * Added code for partial recv() from DHT peers. * Disabled Chat/Disc/Udplayer/tcpstream debug output. * Added Unreachable Check to connMgr. * Added auto reconnect functions to connMgr (#define to disable). * Restructured DHT notify code... much cleaner. * DHT now flags out of date DHT results. * DHT notifies ConnMgr on any results (same or diff). * Added Fns to cleanup old udp connection. * other bugfixes. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@369 b45a01b8-16f6-495d-af2f-9b41ad6348cc
127 lines
3.4 KiB
C++
127 lines
3.4 KiB
C++
/*
|
|
* libretroshare/src/dht: opendhtstr.cc
|
|
*
|
|
* Interface with OpenDHT for RetroShare.
|
|
*
|
|
* Copyright 2007-2008 by Robert Fernie.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "retroshare@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
#include <string>
|
|
#include <sstream>
|
|
|
|
#include "opendhtstr.h"
|
|
#include "b64.h"
|
|
|
|
std::string createHttpHeader(std::string host, uint16_t port, std::string agent, uint32_t length)
|
|
{
|
|
std::ostringstream req;
|
|
|
|
req << "POST / HTTP/1.0\r\n";
|
|
req << "Host: " << host << ":" << port << "\r\n";
|
|
req << "User-Agent: " << agent << "\r\n";
|
|
req << "Content-Type: text/xml\r\n";
|
|
req << "Content-Length: " << length << "\r\n";
|
|
req << "\r\n";
|
|
|
|
return req.str();
|
|
};
|
|
|
|
std::string createHttpHeaderGET(std::string host, uint16_t port, std::string page, std::string agent, uint32_t length)
|
|
{
|
|
std::ostringstream req;
|
|
|
|
req << "GET /" << page << " HTTP/1.0\r\n";
|
|
req << "Host: " << host << ":" << port << "\r\n";
|
|
req << "User-Agent: " << agent << "\r\n";
|
|
//req << "Content-Type: text/xml\r\n";
|
|
//req << "Content-Length: " << length << "\r\n";
|
|
req << "\r\n";
|
|
|
|
return req.str();
|
|
};
|
|
|
|
std::string createOpenDHT_put(std::string key, std::string value, uint32_t ttl, std::string client)
|
|
{
|
|
std::ostringstream req;
|
|
|
|
req << "<?xml version=\"1.0\"?>" << std::endl;
|
|
req << "<methodCall>" << std::endl;
|
|
req << "\t<methodName>put</methodName>" << std::endl;
|
|
req << "\t<params>" << std::endl;
|
|
|
|
req << "\t\t<param><value><base64>";
|
|
req << convertToBase64(key);
|
|
req << "</base64></value></param>" << std::endl;
|
|
|
|
req << "\t\t<param><value><base64>";
|
|
req << convertToBase64(value);
|
|
req << "</base64></value></param>" << std::endl;
|
|
|
|
req << "\t\t<param><value><int>";
|
|
req << ttl;
|
|
req << "</int></value></param>" << std::endl;
|
|
|
|
req << "\t\t<param><value><string>";
|
|
req << client;
|
|
req << "</string></value></param>" << std::endl;
|
|
|
|
req << "\t</params>" << std::endl;
|
|
req << "</methodCall>" << std::endl;
|
|
|
|
return req.str();
|
|
}
|
|
|
|
|
|
std::string createOpenDHT_get(std::string key, uint32_t maxresponse, std::string client)
|
|
{
|
|
std::ostringstream req;
|
|
|
|
req << "<?xml version=\"1.0\"?>" << std::endl;
|
|
req << "<methodCall>" << std::endl;
|
|
req << "\t<methodName>get</methodName>" << std::endl;
|
|
req << "\t<params>" << std::endl;
|
|
|
|
/* key */
|
|
req << "\t\t<param><value><base64>";
|
|
req << convertToBase64(key);
|
|
req << "</base64></value></param>" << std::endl;
|
|
|
|
/* max response */
|
|
req << "\t\t<param><value><int>";
|
|
req << maxresponse;
|
|
req << "</int></value></param>" << std::endl;
|
|
|
|
/* placemark (NULL) */
|
|
req << "\t\t<param><value><base64>";
|
|
req << "</base64></value></param>" << std::endl;
|
|
|
|
req << "\t\t<param><value><string>";
|
|
req << client;
|
|
req << "</string></value></param>" << std::endl;
|
|
|
|
req << "\t</params>" << std::endl;
|
|
req << "</methodCall>" << std::endl;
|
|
|
|
return req.str();
|
|
}
|
|
|
|
|
|
|
|
|