mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
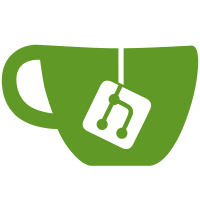
- cleaned include files - changed menu for set the tree is decorated - new check for sort rows by state - saved the state of sort order, hide offline, sort by state and decorated state PopupChatWindow: - when setting "Grab Focus when chat arrives" not checked, the new chat window is opened minimized and flashed in taskbar reworked start private chat and message to friend from PeersDialog and MessengerWindow: - moved method for starting a private chat from PeersDialog and MessengerWindow to PopupChatDialog - moved method for sending a message to a friend from PeersDialog to MessageComposer - removed signal startChat fixed bug in MessengerWindow: - when peer is not online and private chat is not available, the message was send to the wrong peer (the current peer in PeersDialog) git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@3154 b45a01b8-16f6-495d-af2f-9b41ad6348cc
78 lines
2.5 KiB
C++
78 lines
2.5 KiB
C++
/****************************************************************
|
|
* This file is distributed under the following license:
|
|
*
|
|
* Copyright (c) 2006-2007, crypton
|
|
* Copyright (c) 2006, Matt Edman, Justin Hipple
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301, USA.
|
|
****************************************************************/
|
|
|
|
|
|
#ifndef _RETROSHAREWINDOW_H
|
|
#define _RETROSHAREWINDOW_H
|
|
|
|
#include <QString>
|
|
#include <QWidget>
|
|
#include <QVariant>
|
|
#include <QMainWindow>
|
|
#include <gui/settings/rsettings.h>
|
|
|
|
|
|
class RWindow : public QMainWindow
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
/** Default constructor. */
|
|
RWindow(QString name, QWidget *parent = 0, Qt::WFlags flags = 0);
|
|
/** Destructor. */
|
|
~RWindow();
|
|
|
|
/** Associates a shortcut key sequence with a slot. */
|
|
void setShortcut(QString shortcut, const char *slot);
|
|
/** Saves the size and location of the window. */
|
|
void saveWindowState();
|
|
/** Restores the last size and location of the window. */
|
|
void restoreWindowState();
|
|
|
|
/** Gets the saved value of a property associated with this window object.
|
|
* If no value was saved, the default value is returned. */
|
|
QVariant getSetting(QString name, QVariant defaultValue);
|
|
/** Saves a value associated with a setting name for this window object. */
|
|
void saveSetting(QString name, QVariant value);
|
|
|
|
public slots:
|
|
/** Shows or hides this window. */
|
|
virtual void setVisible(bool visible);
|
|
/** Show this window. This method really just exists for subclasses to
|
|
* override, since QMainWindow::show() is non-virtual. */
|
|
virtual void showWindow() { QMainWindow::show(); }
|
|
|
|
signals:
|
|
/** Emitted when a RWindow requests help information on the specified
|
|
* <b>topic</b>. */
|
|
void helpRequested(const QString &topic);
|
|
|
|
protected:
|
|
QString _name; /**< Name associated with this window. */
|
|
|
|
private:
|
|
bool m_bSaveStateOnClose; // is set to true in restoreWindowState
|
|
};
|
|
|
|
#endif
|
|
|