mirror of
https://github.com/RetroShare/RetroShare.git
synced 2025-07-01 17:46:52 -04:00
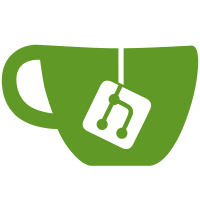
/libretroshare/src/file_sharing/p3filelists.cc👎 In static member function ‘static bool p3FileDatabase::convertPointerToEntryIndex(const void*, p3FileDatabase::EntryIndex&, uint32_t&)’: /libretroshare/src/file_sharing/p3filelists.cc:624: warning: dereferencing type-punned pointer will break strict-aliasing rules [- Wstrict-aliasing] e = EntryIndex( *reinterpret_cast<uint32_t*>(&p) & ENTRY_INDEX_BIT_MASK ) ; /home/phenom/GIT/RetroShare/trunk/libretroshare/src/file_sharing/ p3filelists.cc:625: warning: dereferencing type-punned pointer will break strict-aliasing rules [-Wstrict-aliasing] friend_index = (*reinterpret_cast<uint32_t*>(&p)) >> NB_ENTRY_INDEX_BITS ; /libretroshare/src/gxstrans/p3gxstransitems.h:29: In file included from ../../../trunk/libretroshare/src/gxstrans/p3gxstransitems.h:29:0, /libretroshare/src/gxstrans/p3gxstransitems.cc:19: from ../../../trunk/ libretroshare/src/gxstrans/p3gxstransitems.cc:19: /libretroshare/src/gxstrans/p3gxstransitems.cc👎 In member function ‘virtual void OutgoingRecord_deprecated::serial_process(RsGenericSerializer::SerializeJob, RsGenericSerializer::SerializeContext&)’: /libretroshare/src/serialiser/rstypeserializer.h:61: warning: dereferencing type-punned pointer will break strict-aliasing rules [- Wstrict-aliasing] RsTypeSerializer::serial_process<T>(j, ctx, reinterpret_cast<T&>(I), #I);\ /libretroshare/src/gxstrans/p3gxstransitems.cc:51: in expansion of macro ‘RS_REGISTER_SERIAL_MEMBER_TYPED’ RS_REGISTER_SERIAL_MEMBER_TYPED(clientService, uint16_t); /libretroshare/src/gxstrans/p3gxstransitems.cc👎 In member function ‘virtual void OutgoingRecord::serial_process(RsGenericSerializer::SerializeJob, RsGenericSerializer::SerializeContext&)’: /libretroshare/src/serialiser/rstypeserializer.h:61: warning: dereferencing type-punned pointer will break strict-aliasing rules [- Wstrict-aliasing] RsTypeSerializer::serial_process<T>(j, ctx, reinterpret_cast<T&>(I), #I);\ /libretroshare/src/gxstrans/p3gxstransitems.cc:65: in expansion of macro ‘RS_REGISTER_SERIAL_MEMBER_TYPED’ RS_REGISTER_SERIAL_MEMBER_TYPED(clientService, uint16_t); /retroshare-gui/src/gui/common/RsBanListToolButton.cpp👎 In member function ‘void RsBanListToolButton::applyIp()’: /retroshare-gui/src/gui/common/RsBanListToolButton.cpp:163: warning: ‘list_type’ may be used uninitialized in this function [-Wmaybe- uninitialized] changed = rsBanList->removeIpRange(addr, masked_bytes, list_type);
172 lines
4.1 KiB
C++
172 lines
4.1 KiB
C++
/****************************************************************
|
|
* RetroShare is distributed under the following license:
|
|
*
|
|
* Copyright (C) 2015, RetroShare Team
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor,
|
|
* Boston, MA 02110-1301, USA.
|
|
****************************************************************/
|
|
|
|
#include <QMenu>
|
|
|
|
#include "RsBanListToolButton.h"
|
|
#include "util/RsNetUtil.h"
|
|
|
|
#include <retroshare/rsbanlist.h>
|
|
|
|
/* Use MenuButtonPopup, because the arrow of InstantPopup is too small */
|
|
#define USE_MENUBUTTONPOPUP
|
|
|
|
RsBanListToolButton::RsBanListToolButton(QWidget *parent) :
|
|
QToolButton(parent)
|
|
{
|
|
mList = LIST_WHITELIST;
|
|
mMode = MODE_ADD;
|
|
|
|
setToolButtonStyle(Qt::ToolButtonTextBesideIcon);
|
|
|
|
#ifdef USE_MENUBUTTONPOPUP
|
|
connect(this, SIGNAL(clicked()), this, SLOT(showMenu()));
|
|
#endif
|
|
|
|
updateUi();
|
|
}
|
|
|
|
void RsBanListToolButton::setMode(List list, Mode mode)
|
|
{
|
|
mList = list;
|
|
mMode = mode;
|
|
updateUi();
|
|
}
|
|
|
|
bool RsBanListToolButton::setIpAddress(const QString &ipAddress)
|
|
{
|
|
mIpAddress.clear();
|
|
|
|
if (ipAddress.isEmpty()) {
|
|
updateUi();
|
|
return false;
|
|
}
|
|
|
|
struct sockaddr_storage addr;
|
|
int bytes ;
|
|
|
|
if (!RsNetUtil::parseAddrFromQString(ipAddress, addr, bytes) || bytes != 0) {
|
|
updateUi();
|
|
return false;
|
|
}
|
|
|
|
mIpAddress = ipAddress;
|
|
updateUi();
|
|
|
|
return true;
|
|
}
|
|
|
|
void RsBanListToolButton::updateUi()
|
|
{
|
|
#ifdef USE_MENUBUTTONPOPUP
|
|
setPopupMode(QToolButton::MenuButtonPopup);
|
|
#else
|
|
setPopupMode(QToolButton::InstantPopup);
|
|
#endif
|
|
|
|
switch (mList) {
|
|
case LIST_WHITELIST:
|
|
switch (mMode) {
|
|
case MODE_ADD:
|
|
setText(tr("Add IP to whitelist"));
|
|
break;
|
|
case MODE_REMOVE:
|
|
setText(tr("Remove IP from whitelist"));
|
|
break;
|
|
}
|
|
break;
|
|
case LIST_BLACKLIST:
|
|
switch (mMode) {
|
|
case MODE_ADD:
|
|
setText(tr("Add IP to blacklist"));
|
|
break;
|
|
case MODE_REMOVE:
|
|
setText(tr("Remove IP from blacklist"));
|
|
break;
|
|
}
|
|
break;
|
|
}
|
|
|
|
if (!mIpAddress.isEmpty()) {
|
|
sockaddr_storage addr ;
|
|
int masked_bytes ;
|
|
|
|
if (RsNetUtil::parseAddrFromQString(mIpAddress, addr, masked_bytes)) {
|
|
QMenu *m = new QMenu;
|
|
|
|
m->addAction(QString("%1 %2").arg(tr("Only IP"), RsNetUtil::printAddrRange(addr, 0)), this, SLOT(applyIp()))->setData(0);
|
|
m->addAction(QString("%1 %2").arg(tr("Entire range"), RsNetUtil::printAddrRange(addr, 1)), this, SLOT(applyIp()))->setData(1);
|
|
m->addAction(QString("%1 %2").arg(tr("Entire range"), RsNetUtil::printAddrRange(addr, 2)), this, SLOT(applyIp()))->setData(2);
|
|
|
|
setMenu(m);
|
|
} else {
|
|
setMenu(NULL);
|
|
}
|
|
|
|
setToolTip(mIpAddress);
|
|
} else {
|
|
setMenu(NULL);
|
|
setToolTip("");
|
|
}
|
|
}
|
|
|
|
void RsBanListToolButton::applyIp()
|
|
{
|
|
QAction *action = dynamic_cast<QAction*>(sender());
|
|
if (!action) {
|
|
return;
|
|
}
|
|
|
|
sockaddr_storage addr;
|
|
int masked_bytes;
|
|
|
|
if (!RsNetUtil::parseAddrFromQString(mIpAddress, addr, masked_bytes)) {
|
|
return;
|
|
}
|
|
|
|
uint32_t list_type;
|
|
switch (mList) {
|
|
case LIST_BLACKLIST:
|
|
list_type = RSBANLIST_TYPE_BLACKLIST;
|
|
break;
|
|
case LIST_WHITELIST:
|
|
default:
|
|
list_type = RSBANLIST_TYPE_WHITELIST;
|
|
break;
|
|
}
|
|
|
|
masked_bytes = action->data().toUInt();
|
|
bool changed = false;
|
|
|
|
switch (mMode) {
|
|
case MODE_REMOVE:
|
|
changed = rsBanList->removeIpRange(addr, masked_bytes, list_type);
|
|
break;
|
|
case MODE_ADD:
|
|
default:
|
|
changed = rsBanList->addIpRange(addr, masked_bytes, list_type, "");
|
|
break;
|
|
}
|
|
|
|
if (changed) {
|
|
emit banListChanged(RsNetUtil::printAddrRange(addr, masked_bytes));
|
|
}
|
|
}
|