mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
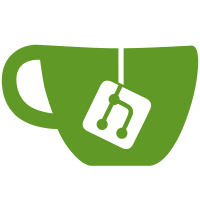
- cleaned source code - added auto refresh Updated english translation git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@6506 b45a01b8-16f6-495d-af2f-9b41ad6348cc
93 lines
2.5 KiB
C++
93 lines
2.5 KiB
C++
/*
|
|
* Retroshare Comment Container
|
|
*
|
|
* Copyright 2012-2012 by Robert Fernie.
|
|
*
|
|
* This library is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Library General Public
|
|
* License Version 2.1 as published by the Free Software Foundation.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Library General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Library General Public
|
|
* License along with this library; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
|
|
* USA.
|
|
*
|
|
* Please report all bugs and problems to "retroshare@lunamutt.com".
|
|
*
|
|
*/
|
|
|
|
#include "gui/gxs/GxsCommentContainer.h"
|
|
#include "gui/gxs/GxsCommentDialog.h"
|
|
|
|
#include <iostream>
|
|
|
|
#define MAX_COMMENT_TITLE 32
|
|
|
|
/****************************************************************
|
|
* GxsCommentContainer
|
|
*
|
|
*/
|
|
|
|
GxsCommentContainer::GxsCommentContainer(QWidget *parent)
|
|
: MainPage(parent)
|
|
{
|
|
ui.setupUi(this);
|
|
|
|
connect(ui.tabWidget, SIGNAL(tabCloseRequested(int)), this, SLOT(tabCloseRequested(int)));
|
|
}
|
|
|
|
void GxsCommentContainer::setup()
|
|
{
|
|
mServiceDialog = createServiceDialog();
|
|
|
|
QString name = getServiceName();
|
|
ui.titleBarLabel->setText(name);
|
|
ui.titleBarPixmap->setPixmap(getServicePixmap());
|
|
|
|
QWidget *widget = dynamic_cast<QWidget *>(mServiceDialog);
|
|
int index = ui.tabWidget->addTab(widget, name);
|
|
ui.tabWidget->hideCloseButton(index);
|
|
}
|
|
|
|
void GxsCommentContainer::commentLoad(const RsGxsGroupId &grpId, const RsGxsMessageId &msgId, const QString &title)
|
|
{
|
|
QString comments = title;
|
|
if (title.length() > MAX_COMMENT_TITLE)
|
|
{
|
|
comments.truncate(MAX_COMMENT_TITLE - 3);
|
|
comments += "...";
|
|
}
|
|
|
|
GxsCommentDialog *commentDialog = new GxsCommentDialog(this, getTokenService(), getCommentService());
|
|
|
|
QWidget *commentHeader = createHeaderWidget(grpId, msgId);
|
|
commentDialog->setCommentHeader(commentHeader);
|
|
|
|
commentDialog->commentLoad(grpId, msgId);
|
|
|
|
ui.tabWidget->addTab(commentDialog, comments);
|
|
}
|
|
|
|
void GxsCommentContainer::tabCloseRequested(int index)
|
|
{
|
|
std::cerr << "GxsCommentContainer::tabCloseRequested(" << index << ")";
|
|
std::cerr << std::endl;
|
|
|
|
if (index != 0)
|
|
{
|
|
QWidget *comments = ui.tabWidget->widget(index);
|
|
ui.tabWidget->removeTab(index);
|
|
delete comments;
|
|
}
|
|
else
|
|
{
|
|
std::cerr << "GxsCommentContainer::tabCloseRequested() Not closing First Tab";
|
|
std::cerr << std::endl;
|
|
}
|
|
}
|