mirror of
https://github.com/RetroShare/RetroShare.git
synced 2024-10-01 02:35:48 -04:00
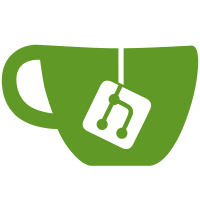
Exports all symbols from RetroShare.exe and import it in the plugins. Removed the direct linking of the libretroshare and libbitdht from the plugins. git-svn-id: http://svn.code.sf.net/p/retroshare/code/trunk@5003 b45a01b8-16f6-495d-af2f-9b41ad6348cc
74 lines
2.1 KiB
C++
74 lines
2.1 KiB
C++
// interface class for p3Voip service
|
|
//
|
|
|
|
#pragma once
|
|
|
|
#include <stdint.h>
|
|
#include <string>
|
|
#include <list>
|
|
#include <vector>
|
|
|
|
class RsVoip ;
|
|
extern RsVoip *rsVoip;
|
|
|
|
static const uint32_t CONFIG_TYPE_VOIP_PLUGIN = 0xe001 ;
|
|
|
|
class RsVoipPongResult
|
|
{
|
|
public:
|
|
RsVoipPongResult()
|
|
:mTS(0), mRTT(0), mOffset(0) { return; }
|
|
|
|
RsVoipPongResult(double ts, double rtt, double offset)
|
|
:mTS(ts), mRTT(rtt), mOffset(offset) { return; }
|
|
|
|
double mTS;
|
|
double mRTT;
|
|
double mOffset;
|
|
};
|
|
|
|
struct RsVoipDataChunk
|
|
{
|
|
void *data ; // create/delete using malloc/free.
|
|
uint32_t size ;
|
|
};
|
|
|
|
class RsVoip
|
|
{
|
|
public:
|
|
virtual int sendVoipHangUpCall(const std::string& peer_id) = 0;
|
|
virtual int sendVoipRinging(const std::string& peer_id) = 0;
|
|
virtual int sendVoipAcceptCall(const std::string& peer_id) = 0;
|
|
|
|
// Sending data. The client keeps the memory ownership and must delete it after calling this.
|
|
virtual int sendVoipData(const std::string& peer_id,const RsVoipDataChunk& chunk) = 0;
|
|
|
|
// The server fill in the data and gives up memory ownership. The client must delete the memory
|
|
// in each chunk once it has been used.
|
|
//
|
|
virtual bool getIncomingData(const std::string& peer_id,std::vector<RsVoipDataChunk>& chunks) = 0;
|
|
|
|
typedef enum { AudioTransmitContinous = 0, AudioTransmitVAD = 1, AudioTransmitPushToTalk = 2 } enumAudioTransmit ;
|
|
|
|
// Config stuff
|
|
|
|
virtual int getVoipATransmit() const = 0 ;
|
|
virtual void setVoipATransmit(int) = 0 ;
|
|
virtual int getVoipVoiceHold() const = 0 ;
|
|
virtual void setVoipVoiceHold(int) = 0 ;
|
|
virtual int getVoipfVADmin() const = 0 ;
|
|
virtual void setVoipfVADmin(int) = 0 ;
|
|
virtual int getVoipfVADmax() const = 0 ;
|
|
virtual void setVoipfVADmax(int) = 0 ;
|
|
virtual int getVoipiNoiseSuppress() const = 0 ;
|
|
virtual void setVoipiNoiseSuppress(int) = 0 ;
|
|
virtual int getVoipiMinLoudness() const = 0 ;
|
|
virtual void setVoipiMinLoudness(int) = 0 ;
|
|
virtual bool getVoipEchoCancel() const = 0 ;
|
|
virtual void setVoipEchoCancel(bool) = 0 ;
|
|
|
|
virtual uint32_t getPongResults(std::string id, int n, std::list<RsVoipPongResult> &results) = 0;
|
|
};
|
|
|
|
|